Bài giảng Công nghệ Java - Chương 10: Handling Mouse and Keyboard Events - SWING components - Lê Nhật Tùng
Topics in This Section
Basic of Event Handling
General asynchronous event-handling strategy
Event-handling options
Handling events with separate listeners
Handling events by implementing interfaces
Handling events with named inner classes
Handling events with anonymous inner classes
The standard AWT listener types
Subtleties with mouse events
Examples
Bạn đang xem 20 trang mẫu của tài liệu "Bài giảng Công nghệ Java - Chương 10: Handling Mouse and Keyboard Events - SWING components - Lê Nhật Tùng", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Công nghệ Java - Chương 10: Handling Mouse and Keyboard Events - SWING components - Lê Nhật Tùng
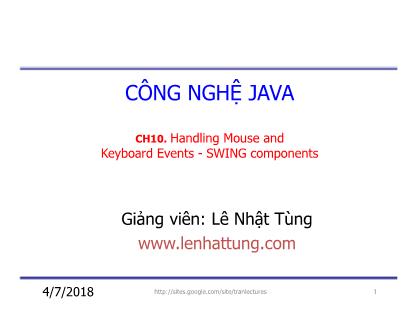
CÔNG NGHỆ JAVA CH10. Handling Mouse and Keyboard Events - SWING components Giảng viên: Lê Nhật Tùng www.lenhattung.com 4/7/2018 1 2 Topics in This Section Basic of Event Handling General asynchronous event-handling strategy Event-handling options Handling events with separate listeners Handling events by implementing interfaces Handling events with named inner classes Handling events with anonymous inner classes The standard AWT listener types Subtleties with mouse events Examples 4/7/2018 3 Basic of Event Handling Every time the user types a character or pushes a mouse button, an event occurs. •Events: Objects that describe what happened •Event sources: The generator of an event •Event handlers: A method that receives an event object, deciphers it, and processes the user’s interaction. 4/7/2018 4 Delegation Model of Event An event can be sent to many event handlers. Event handlers register with components when they are interested in events generated by that component. 4/7/2018 5 A Listener Example public class TestButton { JFrame frame; JButton button; public TestButton() { frame = new JFrame("Test"); button = new JButton("Press Me!"); button.setActionCommand("ButtonPressed"); // register event listener for button button.addActionListener(new ButtonHandler()); frame.add(button, BorderLayout.CENTER); } public void launchFrame() { frame.pack(); frame.setVisible(true); } } 4/7/2018 6 A Listener Example (Contd.) class ButtonHandler implements ActionListener { public void actionPerformed(ActionEvent e) { System.out.println("Action occurred"); System.out.println("Button’s command is: " + e.getActionCommand()); } } 4/7/2018 7 Event Categories Class Hierarchy of GUI Events: 4/7/2018 8 Listener Type Some Events and Their Associated Event Listeners: Act that Results in the Event Listener Type User clicks a button, presses Enter while typing in a text field, or chooses a menu item ActionListener User closes a frame (main window) WindowListener User presses a mouse button while the cursor is over a component MouseListener User moves the mouse over a component MouseMotionListener Component becomes visible ComponentListener Component gets the keyboard focus FocusListener 4/7/2018 9 Listeners •ActionListener Interface: –Has only one method: actionPerformed(ActionEvent) –To detect when the user clicks an onscreen button (or does the keyboard equivalent), a program must have an object that implements the ActionListener interface. –The program must register this object as an action listener on the button (the event source), using the addActionListener() method. –When the user clicks the onscreen button, the button fires an action event. 4/7/2018 10 Listeners (Contd.) MouseListener interface: To detect the mouse clicking, a program must have an object that implements the MouseListener interface. This interface includes several events including mouseEntered, mouseExited, mousePressed, mouseReleased, and mouseClicked. When the user clicks the onscreen button, the button fires an action event. 4/7/2018 11 Listeners (Contd.) Implementing Multiple Interfaces: A class can be declared with Multiple Interfaces by using comma separation: implements MouseListener, MouseMotionListener Listening to Multiple Sources: Multiple listeners cause unrelated parts of a program to react to the same event. The handlers of all registered listeners are called when the event occurs. 4/7/2018 12 General Strategy Determine what type of listener is of interest 11 standard AWT listener types. ActionListener, ItemListener, KeyListener, MouseListener, MouseMotionListener, TextListener, AdjustmentListener, ComponentListener, ContainerListener, FocusListener, WindowListener Define a class of that type Implement interface (KeyListener, MouseListener, ) Extend class (KeyAdapter, MouseAdapter, etc.) Register an object of your listener class with the component comp.addXxxListener(new MyListenerClass()); E.g., addKeyListener, addMouseListener 4/7/2018 Case 1 - Using Separate Listener Classes 4/7/2018 13 14 Separate Listener: Simple Case Listener does not need to call any methods of the window to which it is attached public class MouseClickFrame extends JFrame { public MouseClickFrame(String title) { super(title); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); addMouseListener(new ClickListener()); setSize(300, 400); setVisible(true); } public static void main(String[] args) { new MouseClickFrame("Mouse Click"); } } 4/7/2018 15 Separate Listener: Simple Case class ClickListener extends MouseAdapter { private int radius = 25; public void mousePressed(MouseEvent event) { JFrame comp = (JFrame) event.getSource(); Graphics g = comp.getGraphics(); g.fillOval(event.getX() - radius, event.getY() - radius, 2 * radius, 2 * radius); } } 4/7/2018 16 Separate Listener: Simple Case (Cont') •Register an object of ClickListener for frame •Define a class ClickListener extends MouseAdapter class and override mousePress() method. •General event Handling : –Call event.getSource() to obtain a reference to window or GUI component from which event originated –Cast result to type of interest –Call methods on that reference •get a Graphics object of component (frame) and draw 4/7/2018 17 MouseListener and MouseAdapter public interface MouseListener { public void mouseClicked(MouseEvent e); public void mousePressed(MouseEvent e); public void mouseReleased(MouseEvent e); public void mouseEntered(MouseEvent e); public void mouseExited(MouseEvent e); } public abstract class MouseAdapter implements MouseListener { public void mouseClicked(MouseEvent e) {} public void mousePressed(MouseEvent e) {} public void mouseReleased(MouseEvent e) {} public void mouseEntered(MouseEvent e) {} public void mouseExited(MouseEvent e) {} } 4/7/2018 18 Implementing a Listener Interface class ClickListener implements MouseListener { private int radius = 25; public void mousePressed(MouseEvent event) { JFrame app = (JFrame) event.getSource(); Graphics g = app.getGraphics(); g.fillOval(event.getX() - radius, event.getY() - radius, 2 * radius, 2 * radius); } public void mouseClicked(MouseEvent e) { } public void mouseReleased(MouseEvent e) { } public void mouseEntered(MouseEvent e) { } public void mouseExited(MouseEvent e) {} } 4/7/2018 19 Implementing a Listener Interface public class MouseClickFrame extends JFrame { public MouseClickFrame(String title) { super(title); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); addMouseListener(new ClickListener()); setSize(300, 400); setVisible(true); } public static void main(String[] args) { new MouseClickFrame("Mouse Click"); } } 4/7/2018 20 Adapters vs. Interfaces: Method Signature Errors •What if you goof on the method signature? –public void mousepressed(MouseEvent e) –public void mousePressed() •Interfaces –Compile time error •Adapters –No compile time error, but nothing happens at run time when you press the mouse •Solution for adapters: @Override annotation –Whenever you think you are overriding a method, put @Override on the line above the start of the method. –If that method is not actually overriding an inherited method, you get a compile-time error. 4/7/2018 21 @Override Example class ClickListener extends MouseAdapter { private int radius = 25; @Override public void mousePressed(MouseEvent event) { JFrame comp = (JFrame) event.getSource(); Graphics g = comp.getGraphics(); g.fillOval(event.getX() - radius, event.getY() - radius, 2 * radius, 2 * radius); } } 4/7/2018 Case 2 – Main window implements interface 4/7/2018 22 23 Main window implements interface public class MouseClickFrame extends JFrame implements MouseListener { private int radius = 25; public MouseClickFrame(String title) { super(title); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); addMouseListener(this); setSize(300, 400); setVisible(true); } public void mousePressed(MouseEvent event) { Graphics g = this.getGraphics(); g.fillOval(event.getX() - radius, event.getY() - radius, 2 * radius, 2 * radius); } public void mouseClicked(MouseEvent e) { } public void mouseReleased(MouseEvent e) { } public void mouseEntered(MouseEvent e) { } public void mouseExited(MouseEvent e) { } } Refer to current MouseClickFrame object 4/7/2018 Using Inner Classes (Named & Anonymous) 4/7/2018 24 25 Review of Inner Classes Class can be defined inside another class Methods in the inner class can access all methods and instance variables of surrounding class Even private methods and variables Example public class OuterClass { private int count = ...; public void foo(...) { InnerClass inner = new InnerClass(); inner.bar(); } private class InnerClass { public void bar() { doSomethingWith(count); } } }4/7/2018 26 Case 3: Named Inner Classes public class MouseClickFrame extends JFrame { public MouseClickFrame(String title) { super(title); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); addMouseListener(new ClickListener()) setSize(300, 400); setVisible(true); } private class ClickListener extends MouseAdapter { private int radius = 25; public void mousePressed(MouseEvent event) { Graphics g = getGraphics(); g.fillOval(event.getX()-radius, event.getY()-radius, 2 * radius, 2 * radius); } } } 4/7/2018 27 public class MouseClickFrame extends JFrame { public MouseClickFrame(String title) { super(title); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); addMouseListener(new MouseAdapter() { private int radius = 25; public void mousePressed(MouseEvent event) { Graphics g = getGraphics(); g.fillOval(event.getX()-radius, event.getY()-radius, 2 * radius, 2 * radius); } }); setSize(300, 400); setVisible(true); } } Case 4: Anonymous Inner Classes 4/7/2018 28 Anonymous Inner Classes (other) public class MouseClickFrame extends JFrame { public MouseClickFrame(String title) { super(title); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); MouseListener listener = new MouseAdapter() { private int radius = 25; public void mousePressed(MouseEvent event) { Graphics g = getGraphics(); g.fillOval(event.getX()-radius, event.getY()-radius, 2 * radius, 2 * radius); } }; addMouseListener(listener); setSize(300, 400); setVisible(true); } } 4/7/2018 Summary of Approaches 4/7/2018 29 30 Event Handling Strategies: Pros and Cons Separate Listener Advantages Can extend adapter and thus ignore unused methods Separate class easier to manage Disadvantage Need extra step to call methods in main window Main window that implements interface Advantage No extra steps needed to call methods in main window Disadvantage Must implement methods you might not care about 4/7/2018 31 Event Handling Strategies: Pros and Cons •Named inner class –Advantages •Can extend adapter and thus ignore unused methods •No extra steps needed to call methods in main window –Disadvantage •A bit harder to understand •Anonymous inner class –Advantages •Same as named inner classes •Even shorter –Disadvantage •Much harder to understand 4/7/2018 Event Handler Details and Examples 4/7/2018 32 33 Standard AWT Event Listeners Listener Adapter Class (If Any) Registration Method ActionListener addActionListener AdjustmentListener addAdjustmentListener ComponentListener ComponentAdapter addComponentListener ContainerListener ContainerAdapter addContainerListener FocusListener FocusAdapter addFocusListener ItemListener addItemListener KeyListener KeyAdapter addKeyListener MouseListener MouseAdapter addMouseListener MouseMotionListener MouseMotionAdapter addMouseMotionListener TextListener addTextListener WindowListener WindowAdapter addWindowListener 4/7/2018 34 Standard AWT Event Listeners (Details) ActionListener Handles buttons and a few other actions actionPerformed(ActionEvent event) AdjustmentListener Applies to scrolling adjustmentValueChanged(AdjustmentEvent event) ComponentListener Handles moving/resizing/hiding GUI objects componentResized(ComponentEvent event) componentMoved(ComponentEvent event) componentShown(ComponentEvent event) componentHidden(ComponentEvent event) 4/7/2018 35 Standard Event Listeners (Details cont') ContainerListener Triggered when window adds/removes GUI controls componentAdded(ContainerEvent event) componentRemoved(ContainerEvent event) FocusListener Detects when controls get/lose keyboard focus focusGained(FocusEvent event) focusLost(FocusEvent event) 4/7/2018 36 Standard Event Listeners (Details cont') ItemListener Handles selections in lists, checkboxes, etc. itemStateChanged(ItemEvent event) KeyListener Detects keyboard events keyPressed(KeyEvent event) any key pressed down keyReleased(KeyEvent event) any key released keyTyped(KeyEvent event) key for printable char released 4/7/2018 37 Standard Event Listeners (Details cont') MouseListener Applies to basic mouse events mouseEntered(MouseEvent event) mouseExited(MouseEvent event) mousePressed(MouseEvent event) mouseReleased(MouseEvent event) mouseClicked(MouseEvent event) Release without drag. Do not use this for mousePressed! Applies on release if no movement since press MouseMotionListener Handles mouse movement mouseMoved(MouseEvent event) mouseDragged(MouseEvent event) MouseInputListener Combines MouseListener and MouseMotionListener 4/7/2018 38 Standard Event Listeners (Details cont') TextListener Applies to textfields and text areas textValueChanged(TextEvent event) WindowListener Handles high-level window events windowOpened, windowClosing, windowClosed, windowIconified, windowDeiconified, windowActivated, windowDeactivated windowClosing particularly useful 4/7/2018 39 Example: Simple Whiteboard public class SimpleWhiteboard extends JApplet { protected int lastX=0, lastY=0; public void init() { getContentPane().setBackground(Color.WHITE); getContentPane().setForeground(Color.BLUE); addMouseListener(new PositionRecorder()); addMouseMotionListener(new LineDrawer()); } protected void record(int x, int y) { lastX = x; lastY = y; } 4/7/2018 40 Simple Whiteboard (Continued) private class PositionRecorder extends MouseAdapter { public void mouseEntered(MouseEvent event) { requestFocus(); // Plan ahead for typing record(event.getX(), event.getY()); } public void mousePressed(MouseEvent event) { record(event.getX(), event.getY()); } } private class LineDrawer extends MouseMotionAdapter { public void mouseDragged(MouseEvent event) { int x = event.getX(); int y = event.getY(); Graphics g = getGraphics(); g.drawLine(lastX, lastY, x, y); record(x, y); } } 4/7/2018 41 Simple Whiteboard (Results) 4/7/2018 42 Whiteboard: Adding Keyboard Events public class Whiteboard extends SimpleWhiteboard { protected FontMetrics fm; public void init() { super.init(); Font font = new Font("Serif", Font.BO ... ut"); frame.pack(); frame.setVisible(true); } } 4/7/2018 61 JTextField Example Output 4/7/2018 62 JTextArea JTextArea component is used to accept several lines of text from the user. It implements the scrollable interface to activate scrollbars. JTextArea component can be created using any one of the following constructors: JTextArea() JTextArea(int rows, cols) JTextArea(String text) JTextArea(String text, int rows, int cols) 4/7/2018 63 public class TextAreaApp extends JFrame { public TextAreaAppln(String title) { super(title); setLayout(new FlowLayout()); JLabel lbl = new JLabel("Sample TextArea"); add(lbl); JTextArea txt = new JTextArea(5, 15); txt.setFont(new Font("Serif", Font.ITALIC, 16)); txt.setLineWrap(true); // create croll panel contain text area JScrollPane areaScrollPane = new JScrollPane(txt); add(areaScrollPane); pack(); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } JTextArea Example 4/7/2018 64 JTextArea Example Output 4/7/2018 65 JPasswordField import javax.swing.*; import java.awt.*; import java.awt.event.*; public class PasswordDemo extends Jframe implements ActionListener { JPasswordField txtPassword; public PasswordDemo() { txtPassword = new JPasswordField(12); txtPassword.setEchoChar('*'); JLabel lblPassword = new JLabel("Password : "); lblPassword.setLabelFor(txtPassword); JPanel pnlLeft = new JPanel(); // Add the Label and TextBox to the Panel pnlLeft.add(lblPassword); pnlLeft.add(txtPassword); 4/7/2018 66 JPasswordField JPanel pnlRight = new JPanel(new GridLayout(0,1)); JButton btnOk = new JButton("OK"); JButton btnCancel = new JButton("Cancel"); // Set the command string that will be used for // handling events on the ok button btnOk.setActionCommand("Ok"); btnCancel.setActionCommand("Cancel"); btnOk.addActionListener(this); btnCancel.addActionListener(this); pnlRight.add(btnOk); pnlRight.add(btnCancel); getContentPane().add(pnlLeft, BorderLayout.WEST); getContentPane().add(pnlRight, BorderLayout.CENTER); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setTitle("Password Field Demo"); } 4/7/2018 67 public void actionPerformed(ActionEvent e) { String str = e.getActionCommand(); if ("Ok".equals(str)) { char chPassword[] = txtPassword.getPassword(); String strPassword = new String(chPassword); if (strPassword.trim().equals("pass")) { JOptionPane.showMessageDialog(this, "Correct Password"); } else { JOptionPane.showMessageDialog(this, "Incorrect Password", "Error Message", JOptionPane.ERROR_MESSAGE); } txtPassword.selectAll(); txtPassword.requestFocusInWindow(); } else { System.exit(0); } } JPasswordField (contd) 4/7/2018 68 JPasswordField Contd public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { PasswordDemo frame = new PasswordDemo(); frame.pack(); frame.setVisible(true); } }); } } Output 4/7/2018 69 JButton Buttons trap user action. JButton class descends from javax.swing.AbstractButton class. JButton object consists of a text label and/or image icon, empty area around the text/icon and border. A JButton can be created using: JButton() JButton(Icon icon) JButton(String text) JButton(String text, Icon icon) JButton(Action a) 4/7/2018 70 JButton Example public class ButtonDemo extends JPanel { JButton btnImage1, btnImage2; String message = " "; public ButtonDemo() { // Create icons that display on the buttons ImageIcon btnIcon1 = new ImageIcon("images/red-ball.gif"); ImageIcon btnIcon2 = new ImageIcon("images/cyan-ball.gif"); // Create the buttons btnImage1 = new JButton("First Button", btnIcon1); // Assign hot key for the button label btnImage1.setMnemonic(KeyEvent.VK_F); btnImage1.setActionCommand("first"); btnImage2 = new JButton("Second button", btnIcon2); btnImage2.setMnemonic(KeyEvent.VK_S); btnImage2.setActionCommand("second"); // Register the butttons with the ActionListener ActionListener listener = new ActionHandler(); btnImage1.addActionListener(listener); btnImage2.addActionListener(listener); 4/7/2018 71 JButton Example (contd) add(btnImage1); add(btnImage2); } class ActionHandler implements ActionListener { public void actionPerformed(ActionEvent e) { // If first button clicked if (e.getActionCommand().equals("first")) { message = "You like Red Balls!"; } else if (e.getActionCommand().equals("second")) { message = "You like Cyan Balls!"; } repaint(); } } public void paintComponent(Graphics g) { super.paintComponent(g); g.drawString(message, 100, 150); } } 4/7/2018 72 JButton Example (contd) Output 4/7/2018 73 JCheckbox Checkbox is used to provide the user with a set of options. JCheckBox class has the following constructors: JCheckBox() JCheckBox(Icon icon) JCheckBox(Icon icon, boolean selected) JCheckBox(String text) JCheckBox(String text, boolean selected) JCheckBox(String text, Icon icon) JCheckBox(String text, Icon icon, boolean selected) JCheckBox(Action a) 4/7/2018 74 JCheckbox Example public class CheckBoxDemo extends JPanel { JCheckBox chinButton, glassesButton, hairButton, teethButton; StringBuffer choices; JLabel pictureLabel; public CheckBoxDemo() { // Create the check boxes chinButton = new JCheckBox("Chin"); chinButton.setMnemonic(KeyEvent.VK_C); chinButton.setSelected(true); glassesButton = new JCheckBox("Glasses"); glassesButton.setMnemonic(KeyEvent.VK_G); glassesButton.setSelected(true); hairButton = new JCheckBox("Hair"); hairButton.setMnemonic(KeyEvent.VK_H); hairButton.setSelected(true); teethButton = new JCheckBox("Teeth"); teethButton.setMnemonic(KeyEvent.VK_T); teethButton.setSelected(true); 4/7/2018 75 JCheckbox Example (contd) // Register a listener for the check boxes. CheckBoxListener myListener = new CheckBoxListener(); chinButton.addItemListener(myListener); glassesButton.addItemListener(myListener); hairButton.addItemListener(myListener); teethButton.addItemListener(myListener); // Indicates what's on the geek. choices = new StringBuffer("cght"); // Set up the picture label pictureLabel = new JLabel(new ImageIcon("images/geek/geek-" + choices.toString() + ".gif")); pictureLabel.setToolTipText(choices.toString()); // Put the check boxes in a column in a panel JPanel checkPanel = new JPanel(); checkPanel.setLayout(new GridLayout(0, 1)); checkPanel.add(chinButton); checkPanel.add(glassesButton); checkPanel.add(hairButton); checkPanel.add(teethButton); setLayout(new BorderLayout()); add(checkPanel, BorderLayout.WEST); add(pictureLabel, BorderLayout.CENTER); setBorder(BorderFactory.createEmptyBorder(20, 20, 20, 20)); } 4/7/2018 76 JCheckbox Example (contd) /** Listens to the check boxes. */ class CheckBoxListener implements ItemListener { public void itemStateChanged(ItemEvent e) { int index = 0; char c = '-'; Object source = e.getSource(); if (source == chinButton) { index = 0; c = 'c'; } else if (source == glassesButton) { index = 1; c = 'g'; } else if (source == hairButton) { index = 2; c = 'h'; } else if (source == teethButton) { index = 3; c = 't'; } if (e.getStateChange() == ItemEvent.DESELECTED) c = '-'; choices.setCharAt(index, c); pictureLabel.setIcon(new ImageIcon("images/geek/geek-" + choices.toString() + ".gif")); pictureLabel.setToolTipText(choices.toString()); } } 4/7/2018 77 JCheckbox Example (contd) Output 4/7/2018 78 JRadiobutton A set of radio buttons displays a number of options out of which only one may be selected. A ButtonGroup is used to create a group in Swing. JRadioButton object can be created by using: JRadioButton() JRadioButton(Icon icon) JRadioButton(Icon, boolean selected) JRadioButton(String text) JRadioButton(String text, boolean selected) JRadioButton(String text, Icon icon) JRadioButton(String text, Icon icon, boolean selected) JRadioButton(Action a) 4/7/2018 79 JRadio Example public class RadioButtonDemo extends JPanel { static JFrame frame; static String birdString = "Bird"; static String catString = "Cat"; static String dogString = "Dog"; static String rabbitString = "Rabbit"; static String pigString = "Pig"; JLabel picture; public RadioButtonDemo() { // Create the radio buttons. JRadioButton birdButton = new JRadioButton(birdString); birdButton.setMnemonic(KeyEvent.VK_B); birdButton.setActionCommand(birdString); birdButton.setSelected(true); JRadioButton catButton = new JRadioButton(catString); catButton.setMnemonic(KeyEvent.VK_C); catButton.setActionCommand(catString); JRadioButton dogButton = new JRadioButton(dogString); dogButton.setMnemonic(KeyEvent.VK_D); dogButton.setActionCommand(dogString); 4/7/2018 80 JRadio Example (contd) JRadioButton rabbitButton = new JRadioButton(rabbitString); rabbitButton.setMnemonic(KeyEvent.VK_R); rabbitButton.setActionCommand(rabbitString); JRadioButton pigButton = new JRadioButton(pigString); pigButton.setMnemonic(KeyEvent.VK_P); pigButton.setActionCommand(pigString); // Group the radio buttons. ButtonGroup group = new ButtonGroup(); group.add(birdButton); group.add(catButton); group.add(dogButton); group.add(rabbitButton); group.add(pigButton); // Register a listener for the radio buttons. RadioListener myListener = new RadioListener(); birdButton.addActionListener(myListener); catButton.addActionListener(myListener); dogButton.addActionListener(myListener); rabbitButton.addActionListener(myListener); pigButton.addActionListener(myListener); 4/7/2018 81 JRadio Example (contd) // Set up the picture label picture = new JLabel(new ImageIcon ("images/" + birdString + ".gif")); picture.setPreferredSize(new Dimension(177, 122)); // Put the radio buttons in a column in a panel JPanel radioPanel = new JPanel(); radioPanel.setLayout(new GridLayout(0, 1)); radioPanel.add(birdButton); radioPanel.add(catButton); radioPanel.add(dogButton); radioPanel.add(rabbitButton); radioPanel.add(pigButton); setLayout(new BorderLayout()); add(radioPanel, BorderLayout.WEST); add(picture, BorderLayout.CENTER); setBorder(BorderFactory.createEmptyBorder(20, 20, 20, 20)); } 4/7/2018 82 JRadio Example (contd) /** Listens to the radio buttons. */ class RadioListener implements ActionListener { public void actionPerformed(ActionEvent e) { picture.setIcon(new ImageIcon("images/" + e.getActionCommand() + ".gif")); } } public static void main(String s[]) { frame = new JFrame("RadioButtonDemo"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.getContentPane() .add(new RadioButtonDemo(), BorderLayout.CENTER); frame.pack(); frame.setVisible(true); } } 4/7/2018 83 JRadio Example (contd) Output 4/7/2018 84 JList When the options to choose from is large, the user can be presented with a list to choose . JList component arranges elements one after the other, which can be selected individually or in a group. JList class can display strings as well as icons. JList does not provide support for double clicks. MouseListener can be used to overcome the double click problem. 4/7/2018 85 JList (contd) •public JList() – constructs a JList with an empty model. •public JList(ListModel dataModel) – displays the elements in the specified, non-null list model. •public JList(Object[] listData) – displays the elements of the specified array “listData”. •JList does not support scrolling. To enable scrolling, the following piece of code can be used: JScrollPane myScrollPane = new JScrollPane(); myScrollPane.getViewport().setView(dataList); Or JScrollPane myScrollPane = new JScrollPane(dataList); 4/7/2018 86 JList Example (contd) class AllStars extends JFrame implements ListSelectionListener { String stars[] = {"Antonio Banderas", "Leonardo DiCaprio", "Sandra Bullock", "Hugh Grant", "Julia Roberts" }; JList lstMovieStars = new JList(stars); JLabel lblQuestion = new JLabel("Who is your favorite movie star?"); JTextField txtMovieStar = new JTextField(30); public AllStars(String str) { super(str); JPanel lstPanel = (JPanel) getContentPane(); lstPanel.setLayout(new BorderLayout()); lstPanel.add(lblQuestion, BorderLayout.NORTH); lstMovieStars.setSelectionMode(ListSelectionModel.SINGLE_SELECTION); lstMovieStars.setSelectedIndex(0); // register selection listener lstMovieStars.addListSelectionListener(this); lstMovieStars.setBackground(Color.LIGHT_GRAY); lstMovieStars.setForeground(Color.BLUE); lstPanel.setBackground(Color.WHITE); 4/7/2018 87 JList Example (contd) lstPanel.setForeground(Color.BLACK); lstPanel.add("Center", new JScrollPane(lstMovieStars)); lstPanel.add(txtMovieStar, BorderLayout.SOUTH); } // implement selection listener method public void valueChanged(ListSelectionEvent e) { if (e.getValueIsAdjusting() == false) { if (lstMovieStars.getSelectedIndex() != -1) { txtMovieStar.setText( (String) lstMovieStars.getSelectedValue()); } } } public static void main(String args[]) { AllStars allStars = new AllStars("A sky full of stars!"); allStars.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); allStars.setSize(300, 300); allStars.setVisible(true); } } 4/7/2018 88 JList Example (contd) Output 4/7/2018 89 JComboBox Combination of text field and drop-down list. In Swing, combo box is represented by JComboBox class. public JComboBox() – this constructor creates a JComboBox with a default data model. public JComboBox(ComboBoxModel asModel) – a combo box that takes its items from an existing ComboBoxModel. public JComboBox(Object[] items) – a combo box that contains the elements of the specified array. 4/7/2018 90 JComboBox Example class BestSeller extends JFrame implements ItemListener { String names[] = { "Frederick Forsyth", "John Grisham", "Mary Higgins Clarke", "Patricia Cornwell" }; JComboBox authors = new JComboBox(names); public BestSeller(String str) { super(str); JPanel bestPane = (JPanel) getContentPane(); bestPane.setLayout(new BorderLayout()); authors.addItemListener(this); authors.setBackground(Color.LIGHT_GRAY); authors.setForeground(Color.BLACK); bestPane.setForeground(Color.BLACK); bestPane.add(authors, BorderLayout.PAGE_START); } 4/7/2018 91 JComboBox Example (contd) public void itemStateChanged(ItemEvent e) { if (e.getStateChange() == ItemEvent.SELECTED) { System.out.println((String) e.getItem()); } } public static void main(String args[]) { BestSeller author = new BestSeller("BestSellers!"); author.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); author.setSize(200, 200); author.setVisible(true); } } 4/7/2018 92 JComboBox Example (contd) Output 4/7/2018 93 Summary The Java Foundation Classes (JFC) are developed as an extension to Abstract Windows Toolkit (AWT), to overcome the shortcomings of AWT. Swing is a set of classes under the JFC that provide lightweight visual components and enable creation of an attractive GUI. Swing Applets provide support for assistive technologies and a RootPane (and thus support for adding menubar). With Swing, most of the components can display text as well as images.
File đính kèm:
bai_giang_cong_nghe_java_chuong_10_handling_mouse_and_keyboa.pdf