Bài giảng Công nghệ Java - Chương 14: Java Socket Programming - Trần Quang Diệu
Java Sockets Programming
• The package java.net provides support for
sockets programming (and more).
• Typically you import everything defined in this
package with:
import java.net.*;
Bạn đang xem 20 trang mẫu của tài liệu "Bài giảng Công nghệ Java - Chương 14: Java Socket Programming - Trần Quang Diệu", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Công nghệ Java - Chương 14: Java Socket Programming - Trần Quang Diệu
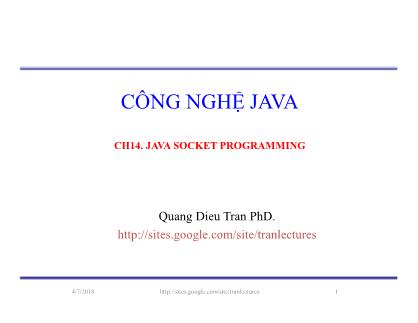
4/7/2018 1 CÔNG NGHỆ JAVA CH14. JAVA SOCKET PROGRAMMING Quang Dieu Tran PhD. Java Sockets Programming • The package java.net provides support for sockets programming (and more). • Typically you import everything defined in this package with: import java.net.*; 24/7/2018 Classes InetAddress Socket ServerSocket DatagramSocket DatagramPacket 34/7/2018 InetAddress class • static methods you can use to create new InetAddress objects. – getByName(String host) – getAllByName(String host) – getLocalHost() InetAddress x = InetAddress.getByName( “cse.unr.edu”); Throws UnknownHostException 44/7/2018 Sample Code: Lookup.java • Uses InetAddress class to lookup hostnames found on command line. > java Lookup cse.unr.edu www.yahoo.com cse.unr.edu:134.197.40.9 www.yahoo.com:209.131.36.158 54/7/2018 try { InetAddress a = InetAddress.getByName(hostname); System.out.println(hostname + ":" + a.getHostAddress()); } catch (UnknownHostException e) { System.out.println("No address found for " + hostname); } es 4/7/2018 6 Socket class • Corresponds to active TCP sockets only! – client sockets – socket returned by accept(); • Passive sockets are supported by a different class: – ServerSocket • UDP sockets are supported by – DatagramSocket 74/7/2018 JAVA TCP Sockets • java.net.Socket – Implements client sockets (also called just “sockets”). – An endpoint for communication between two machines. – Constructor and Methods • Socket(String host, int port): Creates a stream socket and connects it to the specified port number on the named host. • InputStream getInputStream() • OutputStream getOutputStream() • close() • java.net.ServerSocket – Implements server sockets. – Waits for requests to come in over the network. – Performs some operation based on the request. – Constructor and Methods • ServerSocket(int port) • Socket Accept(): Listens for a connection to be made to this socket and accepts it. This method blocks until a connection is made. 84/7/2018 Sockets 9 Client socket, welcoming socket (passive) and connection socket (active) 4/7/2018 Socket Constructors • Constructor creates a TCP connection to a named TCP server. – There are a number of constructors: Socket(InetAddress server, int port); Socket(InetAddress server, int port, InetAddress local, int localport); Socket(String hostname, int port); 104/7/2018 Socket Methods void close(); InetAddress getInetAddress(); InetAddress getLocalAddress(); InputStream getInputStream(); OutputStream getOutputStream(); • Lots more (setting/getting socket options, partial close, etc.) 114/7/2018 Socket I/O • Socket I/O is based on the Java I/O support – in the package java.io • InputStream and OutputStream are abstract classes – common operations defined for all kinds of InputStreams, OutputStreams 124/7/2018 InputStream Basics // reads some number of bytes and // puts in buffer array b int read(byte[] b); // reads up to len bytes int read(byte[] b, int off, int len); Both methods can throw IOException. Both return –1 on EOF. 134/7/2018 OutputStream Basics // writes b.length bytes void write(byte[] b); // writes len bytes starting // at offset off void write(byte[] b, int off, int len); Both methods can throw IOException. 144/7/2018 ServerSocket Class (TCP Passive Socket) • Constructors: ServerSocket(int port); ServerSocket(int port, int backlog); ServerSocket(int port, int backlog, InetAddress bindAddr); 154/7/2018 ServerSocket Methods Socket accept(); void close(); InetAddress getInetAddress(); int getLocalPort(); throw IOException, SecurityException 164/7/2018 Socket programming with TCP Example client-server app: • client reads line from standard input (inFromUser stream) , sends to server via socket (outToServer stream) • server reads line from socket • server converts line to uppercase, sends back to client • client reads, prints modified line from socket (inFromServer stream) 17 o u tT o S e rv e r to network from network in F ro m S e rv e r in F ro m U se r keyboard monitor Process clientSocket input stream input stream output stream TCP socket Input stream: sequence of bytes into processoutput stream: sequence of bytes out of process Client process client TCP socket 4/7/2018 Client/server socket interaction: TCP 18 wait for incoming connection request connectionSocket = welcomeSocket.accept() create socket, port=x, for incoming request: welcomeSocket = ServerSocket() create socket, connect to hostid, port=x clientSocket = Socket() close connectionSocket read reply from clientSocket close clientSocket Server (running on hostid) Client send request using clientSocketread request from connectionSocket write reply to connectionSocket TCP connection setup 4/7/2018 TCPClient.java import java.io.*; import java.net.*; class TCPClient { public static void main(String argv[]) throws Exception { String sentence; String modifiedSentence; BufferedReader inFromUser = new BufferedReader(new InputStreamReader(System.in)); Socket clientSocket = new Socket("hostname", 6789); DataOutputStream outToServer = new DataOutputStream(clientSocket.getOutputStream()); 4/7/2018 19 TCPClient.java BufferedReader inFromServer = new BufferedReader(new InputStreamReader(clientSocket.getInputStream())); sentence = inFromUser.readLine(); outToServer.writeBytes(sentence + '\n'); modifiedSentence = inFromServer.readLine(); System.out.println("FROM SERVER: " + modifiedSentence); clientSocket.close(); } } 204/7/2018 TCPServer.java import java.io.*; import java.net.*; class TCPServer { public static void main(String argv[]) throws Exception { String clientSentence; String capitalizedSentence; ServerSocket welcomeSocket = new ServerSocket(6789); while(true) { Socket connectionSocket = welcomeSocket.accept(); BufferedReader inFromClient = new BufferedReader(new InputStreamReader(connectionSocket.getInputStream())); 4/7/2018 21 TCPServer.java DataOutputStream outToClient = new DataOutputStream(connectionSocket.getOutputStream()); clientSentence = inFromClient.readLine(); capitalizedSentence = clientSentence.toUpperCase() + '\n'; outToClient.writeBytes(capitalizedSentence); } } } 224/7/2018 Sample Echo Server TCPEchoServer.java Simple TCP Echo server. Based on code from: TCP/IP Sockets in Java 234/7/2018 UDP Sockets • DatagramSocket class • DatagramPacket class needed to specify the payload – incoming or outgoing 244/7/2018 Socket Programming with UDP • UDP – Connectionless and unreliable service. – There isn’t an initial handshaking phase. – Doesn’t have a pipe. – transmitted data may be received out of order, or lost • Socket Programming with UDP – No need for a welcoming socket. – No streams are attached to the sockets. – the sending hosts creates “packets” by attaching the IP destination address and port number to each batch of bytes. – The receiving process must unravel to received packet to obtain the packet’s information bytes. 254/7/2018 JAVA UDP Sockets • In Package java.net – java.net.DatagramSocket • A socket for sending and receiving datagram packets. • Constructor and Methods – DatagramSocket(int port): Constructs a datagram socket and binds it to the specified port on the local host machine. – void receive( DatagramPacket p) – void send( DatagramPacket p) – void close() 264/7/2018 DatagramSocket Constructors DatagramSocket(); DatagramSocket(int port); DatagramSocket(int port, InetAddress a); All can throw SocketException or SecurityException 274/7/2018 Datagram Methods void connect(InetAddress, int port); void close(); void receive(DatagramPacket p); void send(DatagramPacket p); Lots more! 284/7/2018 Datagram Packet • Contain the payload – (a byte array • Can also be used to specify the destination address – when not using connected mode UDP 294/7/2018 DatagramPacket Constructors For receiving: DatagramPacket( byte[] buf, int len); For sending: DatagramPacket( byte[] buf, int len InetAddress a, int port); 304/7/2018 DatagramPacket methods byte[] getData(); void setData(byte[] buf); void setAddress(InetAddress a); void setPort(int port); InetAddress getAddress(); int getPort(); 314/7/2018 Example: Java client (UDP) 32 se n d P a ck e t to network from network re ce iv e P a c k e t in F ro m U se r keyboard monitor Process clientSocket UDP packet input stream UDP packet UDP socket Output: sends packet (TCP sent “byte stream”) Input: receives packet (TCP received “byte stream”) Client process client UDP socket 4/7/2018 Client/server socket interaction: UDP 33 close clientSocket Server (running on hostid) read reply from clientSocket create socket, clientSocket = DatagramSocket() Client Create, address (hostid, port=x, send datagram request using clientSocket create socket, port=x, for incoming request: serverSocket = DatagramSocket() read request from serverSocket write reply to serverSocket specifying client host address, port umber 4/7/2018 UDPClient.java import java.io.*; import java.net.*; class UDPClient { public static void main(String args[]) throws Exception { BufferedReader inFromUser = new BufferedReader(new InputStreamReader(System.in)); DatagramSocket clientSocket = new DatagramSocket(); InetAddress IPAddress = InetAddress.getByName("hostname"); byte[] sendData = new byte[1024]; byte[] receiveData = new byte[1024]; String sentence = inFromUser.readLine(); sendData = sentence.getBytes(); 4/7/2018 34 UDPClient.java DatagramPacket sendPacket = new DatagramPacket(sendData, sendData.length, IPAddress, 9876); clientSocket.send(sendPacket); DatagramPacket receivePacket = new DatagramPacket(receiveData, receiveData.length); clientSocket.receive(receivePacket); String modifiedSentence = new String(receivePacket.getData()); System.out.println("FROM SERVER:" + modifiedSentence); clientSocket.close(); } } 354/7/2018 UDPServer.java import java.io.*; import java.net.*; class UDPServer { public static void main(String args[]) throws Exception { DatagramSocket serverSocket = new DatagramSocket(9876); byte[] receiveData = new byte[1024]; byte[] sendData = new byte[1024]; while(true) { DatagramPacket receivePacket = new DatagramPacket(receiveData, receiveData.length); serverSocket.receive(receivePacket); String sentence = new String(receivePacket.getData()); 4/7/2018 36 UDPServer.java InetAddress IPAddress = receivePacket.getAddress(); int port = receivePacket.getPort(); String capitalizedSentence = sentence.toUpperCase(); sendData = capitalizedSentence.getBytes(); DatagramPacket sendPacket = new DatagramPacket(sendData, sendData.length, IPAddress, port); serverSocket.send(sendPacket); } } } 374/7/2018 Sample UDP code UDPEchoServer.java Simple UDP Echo server. Test using nc as the client (netcat): > nc –u hostname port 384/7/2018 Socket functional calls • socket (): Create a socket • bind(): bind a socket to a local IP address and port # • listen(): passively waiting for connections • connect(): initiating connection to another socket • accept(): accept a new connection • Write(): write data to a socket • Read(): read data from a socket • sendto(): send a datagram to another UDP socket • recvfrom(): read a datagram from a UDP socket • close(): close a socket (tear down the connection) 394/7/2018 Java URL Class • Represents a Uniform Resource Locator – scheme (protocol) – hostname – port – path – query string 404/7/2018 Parsing • You can use a URL object as a parser: URL u = new URL(“”); System.out.println(“Proto:” + u.getProtocol()); System.out.println(“File:” + u.getFile()); 414/7/2018 URL construction • You can also build a URL by setting each part individually: URL u = new URL(“http”, www.cs.unr.edu,80,”/~mgunes/”); System.out.println(“URL:” + u.toExternalForm()); System.out.println(“URL: “ + u); 424/7/2018 Retrieving URL contents • URL objects can retrieve the documents they refer to! – actually this depends on the protocol part of the URL. – HTTP is supported – File is supported (“file://c:\foo.html”) – You can get “Protocol Handlers” for other protocols. • There are a number of ways to do this: Object getContent(); InputStream openStream(); URLConnection openConnection(); 434/7/2018 Getting Header Information • There are methods that return information extracted from response headers: String getContentType(); String getContentLength(); long getLastModified(); 444/7/2018 URLConnection • Represents the connection (not the URL itself). • More control than URL – can write to the connection (send POST data). – can set request headers. • Closely tied to HTTP 454/7/2018
File đính kèm:
bai_giang_cong_nghe_java_chuong_14_java_socket_programming_t.pdf