Bài giảng Công nghệ Java - Chương 9: GUI Programming & Swing - Trần Quang Diệu
What is a SWING
2
• Swing is the next-generation GUI toolkit that Sun
Microsystems created to enable enterprise
development in Java.
• Swing is part of a larger family of Java products
known as the Java Foundation Classes ( JFC)
• The Swing package was first available as an add-on
to JDK 1.1.
– Prior to the introduction of the Swing package, the
Abstract Window Toolkit (AWT) components provided all
the UI components in the JDK 1.0 and 1.1 platforms.
Bạn đang xem 20 trang mẫu của tài liệu "Bài giảng Công nghệ Java - Chương 9: GUI Programming & Swing - Trần Quang Diệu", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Công nghệ Java - Chương 9: GUI Programming & Swing - Trần Quang Diệu
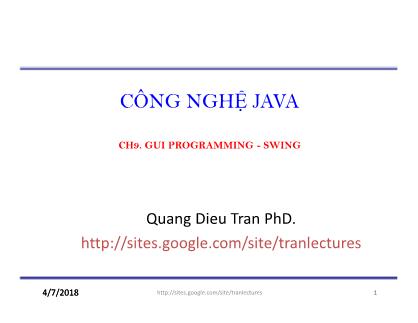
CÔNG NGHỆ JAVA CH9. GUI PROGRAMMING - SWING Quang Dieu Tran PhD. 14/7/2018 What is a SWING 2 • Swing is the next-generation GUI toolkit that Sun Microsystems created to enable enterprise development in Java. • Swing is part of a larger family of Java products known as the Java Foundation Classes ( JFC) • The Swing package was first available as an add-on to JDK 1.1. – Prior to the introduction of the Swing package, the Abstract Window Toolkit (AWT) components provided all the UI components in the JDK 1.0 and 1.1 platforms. 4/7/2018 Swing Toolkit 3 • 100% Java implementation of components • Pluggable Look & Feel – customizable for different environments, or – use Java Look & Feel in every environment • Lightweight components – no separate (child) windows for components – allows more variation on component structure – makes Look & Feel possible • Three parts – component set (subclasses of JComponent) – support classes – interfaces 4/7/2018 Other APIs 44/7/2018 Swing Overview 5 • Swing Components and the Containment Hierarchy – Swing provides many standard GUI components such as buttons, lists, menus, and text areas, windows, tool bars. • Layout Management – Containers use layout managers to determine the size and position of the components they contain. • Event Handling – Event handling is how programs respond to external events, such as the user pressing a mouse button. • Painting – Painting means drawing the component on-screen. – it's easy to customize a component's painting. • More Swing Features and Concepts – include support for icons, actions, Pluggable Look & Feel technology, 4/7/2018 import java.awt.*; import javax.swing.*; public class HelloAppTest { public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { HelloFrame frame = new HelloFrame("Hello World App"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }); } } class HelloFrame extends JFrame { public HelloFrame(String title) { super(title); JLabel label = new JLabel("Hello World!"); getContentPane().add(label); setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT); } public static final int DEFAULT_WIDTH = 300; public static final int DEFAULT_HEIGHT = 200; } Example 64/7/2018 Walk through this program 7 • What to import – You need to import both java.awt.* and javax.swing.* to get all the basic classes. import java.awt.*; import javax.swing.*; ... HelloFrame frame = new HelloFrame("Hello World App"); • Create Frame –Creating a JFrame gives you a "top level" container. •A top-level window –The string passed to the constructor is the title of the window. 4/7/2018 Adding component 8 • The Content Pane – components must add to the frame’s content cane. – Call getContentPane() to get the content pane. • Adding content – Create another Swing component. • In this case, a label is created with the text "Hello, World!". – Call add() with the component as a parameter to add it to the frame’s content pane. JLabel hello = new JLabel("Hello, world!"); frame.getContentPane().add(hello); 4/7/2018 Resizing and showing 9 • setSize(width, height) • pack() frame to the minimum size needed to display the items in the content pane. • Calling setVisible(true) will show the JFrame. – You must set the size of the frame (directly or with pack()) before you show the frame. frame.pack(); frame.setVisible(true); 4/7/2018 Swing Components 104/7/2018 Swing Components Example 11 • SwingApplication example creates four commonly used Swing components: – a frame, or main window (JFrame) – a panel, sometimes called a pane (JPanel) – a button (JButton) – a label (JLabel) frame panel button label 4/7/2018 Swing Container 12 • The frame (JFrame) is a top-level container. – Provide a place for other components to paint themselves. – The other commonly used top-level containers are dialogs (JDialog) and applets (JApplet). • The panel (JPanel) is an intermediate container. – Used to group small lightweight components together – Other intermediate Swing containers, such as scroll panes (JScrollPane) and tabbed panes (JTabbedPane), typically play a more visible, interactive role in a program's GUI. 4/7/2018 Swing Atomic Components 13 • The button and label are atomic components – components that exist as self-sufficient entities that present bits of information to the user. • Often, atomic components also get input from the user. • The Swing API provides many atomic components – Button (JButton) – Combo boxes (JComboBox), – Text fields (JTextField), – Tables (JTable). – 4/7/2018 The Containment Hierarchy 14 • A diagram of the containment hierarchy shows each container created or used by the program, along with the components it contains. SwingApplication Even the simplest Swing program has multiple levels in its containment hierarchy. The root of the containment hierarchy is always a top- level container. 4/7/2018 Swing Components 4/7/2018 15 Top-Level Containers 16 JFrame JApplet JDialog 4/7/2018 General-Purpose Containers 17 • Intermediate containers that can be used under many different circumstances. JPanel JScrollPane JTabbedPane JSplitPane JToolBar JOptionPane 4/7/2018 Special-Purpose Containers 18 JInternalFrame JLayeredPane 4/7/2018 Basic Controls - Buttons 19 • Basic Controls: Atomic components that exist primarily to get input from the user; they generally also show simple state. 4/7/2018 Basic Controls - MENUS 20 JMenuBar 4/7/2018 Basic Controls - Others 21 JComboBox JList JScrollBar JSlider 4/7/2018 Uneditable Information Displays 22 • Atomic components that exist solely to give the user information. JLabel JTooltip JProgressBar JImageIcon 4/7/2018 Editable Displays of Formatted Information 23 •Atomic components that display highly formatted information that (if you choose) can be edited by the user. JColorChooser JFileChooser JTable JTextArea JTree JTextField 4/7/20184/7/2018 23 244/7/2018 Layout Managers Arranging Elements in Windows Layout Management 25 • Layout management is the process of determining the size and position of components. – By default, each container has a layout manager - an object that performs layout management for the components within the container. – Components can provide size and alignment hints to layout managers, but layout managers have the final say on the size and position of those components. • The Java platform supplies commonly used layout managers: – FlowLayout, BorderLayout, BoxLayout, CardLayout GridLayout, and GridBagLayout. 4/7/2018 Applications of Layout Managers 26 • Each layout manager has its own particular use –For displaying a few components of same size in rows and columns, the GridLayoutwould be appropriate –To display a component in maximum possible space, a choice between BorderLayout and GridBagLayout has to be made. • How to set layouts? –When a container is first created, it uses its default layout manager. • Default layout of an frame is BorderLayout –A new layout manager can be set using the setLayout() method. 4/7/2018 Layout Manager – FlowLayout 27 • Default layout for Panel, JPanel, and Applet • Resizes components to their preferred size • Places components in rows left to right, starting new rows if necessary. • Rows are centered by default 4/7/2018 FlowLayout - Example 28 public class FlowLayoutFrame extends JFrame{ public FlowLayoutFrame(String title) { super(title); this.setLayout(new FlowLayout()); this.add(new JButton("Button 1")); this.add(new JButton("2")); this.add(new JButton("Button 3")); this.add(new JButton("Long-Named Button 4")); this.add(new JButton("Button 5")); this.pack(); this.setVisible(true); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } 4/7/2018 FlowLayout constructors 29 • public FlowLayout() • public FlowLayout(int alignment) – The alignment must have the value FlowLayout.LEFT, FlowLayout.CENTER, or FlowLayout.RIGHT. • public FlowLayout(int alignment, int horizontalGap, int verticalGap) – The horizontalGap and verticalGap specify the number of pixels to put between components. If you don't specify a gap value, FlowLayout uses 5 for the default gap value. 4/7/2018 Layout Manager – BorderLayout 30 • Is the default layout manager of the content pane of every JFrame. • A BorderLayout has five areas available to hold components: north, south, east, west, and center. 4/7/2018 BorderLayout - Example 31 public class BorderLayoutFrame extends JFrame{ public BorderLayoutFrame(String title) { super(title); // Use the content pane's default BorderLayout. setLayout(new BorderLayout(2, 2)); add(new JButton("Button 1 (NORTH)"), BorderLayout.NORTH); add(new JButton("Button 2 (CENTER)"), BorderLayout.CENTER); add(new JButton("Button 3 (WEST)"), BorderLayout.WEST); add(new JButton("Long-Named Button 4 (SOUTH)"), BorderLayout.SOUTH); add(new JButton("Button 5 (EAST)"), BorderLayout.EAST); pack(); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } 4/7/2018 The BorderLayout API 32 • Constructors – BorderLayout() • Border layout with no gaps between components – BorderLayout(int hGap, int vGap) • Border layout with the specified empty pixels between regions • Adding Components – add(component, BorderLayout.REGION) – Always specify the region in which to add the component: • CENTER is the default • to set the horizontal and vertical gaps, use: – setHgap(int) – setVgap(int) 4/7/2018 Layout Manager – BoxLayout 33 • The BoxLayout class puts components in a single row or column. • It respects the components' requested maximum sizes, and also lets you align components. 4/7/2018 Layout Manager – CardLayout 34 • The CardLayout class lets you implement an area that contains different components at different times. • A CardLayout is often controlled by a combo box, with the state of the combo box determining which panel (group of components) the CardLayout displays. • Tabbed panes are intermediate Swing containers that provide similar functionality. 4/7/2018 CardLayout 35 • Stacks components on top of each other, displaying the top one • Associates a name with each component in window Panel cardPanel; CardLayout layout = new CardLayout(); cardPanel.setLayout(layout); ... cardPanel.add("Card 1", component1); cardPanel.add("Card 2", component2); ... layout.show(cardPanel, "Card 1"); layout.first(cardPanel); layout.next(cardPanel); 4/7/2018 CardLayout, Example 364/7/2018 GridLayout 37 • Divides window into equal-sized rectangles based upon the number of rows and columns specified – Items placed into cells left-to-right, top-to-bottom, based on the order added to the container – Ignores the preferred size of the component; each component is resized to fit into its grid cell • Too few components results in blank cells • Too many components results in extra columns 4/7/2018 GridLayout 38 Container contentPane = getContentPane(); contentPane.setLayout(new GridLayout(0, 2)); contentPane.add(new JButton("Button 1")); contentPane.add(new JButton("2")); contentPane.add(new JButton("Button 3")); contentPane.add(new JButton("Long-Named Button 4")); contentPane.add(new JButton("Button 5")); The constructor tells the GridLayout class to create an instance that has two columns and as many rows as necessary. 4/7/2018 The GridLayout Constructors 39 • GridLayout() – Creates a single row with one column allocated per component • GridLayout(int rows, int cols) – Divides the window into the specified number of rows and columns – Either rows or cols (but not both) can be zero • GridLayout(int rows, int cols, int hGap, int vGap) – Uses the specified gaps between cells 4/7/2018 GridBagLayout 40 • is the most sophisticated, flexible layout manager. • It aligns components by placing them within a grid of cells, allowing some components to span more than one cell. – The grid rows and columns aren't necessarily all the same size. • Each component managed by a grid bag layout is associated with an instance of GridBagConstraints – The GridBagConstraints specifies: • How the component is laid out in the display area • In which cell the component starts and ends • How the component stretches when extra room is available • Alignment in cells 4/7/2018 GridBagLayout: Basic Steps 41 • Set the layout, saving a reference to it GridBagLayout layout = new GridBagLayout(); setLayout(layout); • Allocate a GridBagConstraints object GridBagConstraints constraints = new GridBagConstraints(); • Set up the GridBagConstraints for component 1 constraints.gridx = x1; constraints.gridy = y1; constraints.gridwidth = width1; constraints.gridheight = height1; • Add component 1 to the window, including constraints add(component1, constraints); • Repeat the last two steps for each remaining component 4/7/2018 GridBagConstraints Fields 42 • gridx, gridy –Specifies the column and row positions of the top-left –corner of the component to be added. –Upper left of grid is located at s(0,0) –Set to GridBagConstraints.RELATIVE to auto- increment row/column GridBagConstraints constraints = new GridBagConstraints(); constraints.gridx = GridBagConstraints.RELATIVE; container.add(new Button("one"), constraints); container.add(new Button("two"), constraints); 4/7/2018 GridBagConstraints Fields 43 • gridwidth, gridheight – Determine how many columns and rows the component occupies constraints.gridwidth = 3; – GridBagConstraints.REMAINDER lets the component take up the remainder of the row/column • weightx, weighty – Specifies how much the cell will stretch in the x or y direction if space is left over constraints.weightx = 1.0; – Constraint affects the cell, not the component (use fill) – Use a value of 0.0 for no grow or shrink beyond its initial size in that direction – Values are relative, not absolute 4/7/2018 GridBagConstraints Fields 44 • fill – Specifies what to do to an element that is smaller than the cell size constraints.fill = GridBagConstraints.VERTICAL; – The size of row/column is determined by the widest/tallest element in it – Can be NONE, HORIZONTAL, VERTICAL, or BOTH • anchor – If the fill is set to GridBagConstraints.NONE, then the anchor field determines where the component is placed constraints.anchor = GridBagConstraints.NORTHEAST; – Can be NORTH, EAST, SOUTH, WEST, NORTHEAST, NORTHWEST, SOUTHEAST, or SOUTHWEST 4/7/2018 GridBagLayout: Example 454/7/2018 GridBagLayout: Example 46 public GridBagTest() { setLayout(new GridBagLayout()); textArea = new JTextArea(12, 40); // 12 rows, 40 cols btnSaveAs = new JButton("Save As"); txtFile = new JTextField("C:\\Document.txt"); btbOk = new JButton("OK"); btnExit = new JButton("Exit"); gbc = new GridBagConstraints(); // Text Area. gbc.gridx = 0; gbc.gridy = 0; gbc.gridwidth = GridBagConstraints.REMAINDER; gbc.gridheight = 1; gbc.weightx = 1.0; gbc.weighty = 1.0; gbc.fill = GridBagConstraints.BOTH; gbc.insets = new Insets(2, 2, 2, 2); // t,l,b,r add(textArea, gbc); 4/7/2018 GridBagLayout: Example (con't) 47 // Save As Button. gbc.gridx = 0; gbc.gridy = 1; gbc.gridwidth = 1; gbc.gridheight = 1; gbc.weightx = 0.0; gbc.weighty = 0.0; gbc.fill = GridBagConstraints.VERTICAL; add(btnSaveAs, gbc); // Filename Input (Textfield). gbc.gridx = 1; gbc.gridwidth = GridBagConstraints.REMAINDER; gbc.gridheight = 1; gbc.weightx = 1.0; gbc.weighty = 0.0; gbc.fill = GridBagConstraints.BOTH; add(txtFile, gbc); 4/7/2018 GridBagLayout: Example (con't) 48 // OK Button. gbc.gridx = 2; gbc.gridy++; gbc.gridwidth = 1; gbc.gridheight = 1; gbc.weightx = 0.0; gbc.weighty = 0.0; gbc.fill = GridBagConstraints.NONE; add(btnOk, gbc); // Exit Button. gbc.gridx = 3; gbc.gridwidth = 1; gbc.gridheight = 1; gbc.weightx = 0.0; gbc.weighty = 0.0; gbc.fill = GridBagConstraints.NONE; add(btnExit, gbc); // Filler so Column 1 has nonzero width. Component filler = Box.createRigidArea(new Dimension(1, 1)); gbc.gridx = 1; gbc.weightx = 1.0; add(filler, gbc); } 4/7/2018 GridBagLayout: Result 494/7/2018 Strategies for Using Layout Managers 504/7/2018 Absolute layout – Disabling the Layout Manager 51 • Behavior –If the layout is set to null, then components must be sized and positioned by hand – Positioning components –component.setSize(width, height) –component.setLocation(left, top) or –component.setBounds(left, top, width, height) 4/7/2018 Absolute layout: Example 52 setLayout(null); Button b1 = new Button("Button 1"); Button b2 = new Button("Button 2"); ... b1.setBounds(0, 0, 150, 50); b2.setBounds(150, 0, 75, 50); ... add(b1); add(b2); ... 4/7/2018 Using Layout Managers Effectively 53 • Use nested containers – Rather than struggling to fit your design in a single layout, try dividing the design into sections – Let each section be a panel with its own layout manager • Turn off the layout manager for some containers • Adjust the empty space around components – Change the space allocated by the layout manager – Override insets in the Container – Use a Canvas or a Box as an invisible spacer 4/7/2018 Nested Containers: Example 544/7/2018 Nested Containers: Example 55 public NestedLayout() { setLayout(new BorderLayout(2, 2)); setBorder(BorderFactory.createEtchedBorder()); textArea = new JTextArea(12, 40); // 12 rows, 40 cols bSaveAs = new JButton("Save As"); fileField = new JTextField("C:\\Document.txt"); bOk = new JButton("OK"); bExit = new JButton("Exit"); add(textArea, BorderLayout.CENTER); // Set up buttons and textfield in bottom panel. JPanel bottomPanel = new JPanel(); bottomPanel.setLayout(new GridLayout(2, 1)); 4/7/2018 Nested Containers: Example 56 JPanel subPanel1 = new JPanel(); JPanel subPanel2 = new JPanel(); subPanel1.setLayout(new BorderLayout()); subPanel2.setLayout( new FlowLayout(FlowLayout.RIGHT, 2, 2)); subPanel1.add(bSaveAs, BorderLayout.WEST); subPanel1.add(fileField, BorderLayout.CENTER); subPanel2.add(bOk); subPanel2.add(bExit); bottomPanel.add(subPanel1); bottomPanel.add(subPanel2); add(bottomPanel, BorderLayout.SOUTH); } 4/7/2018 Nested Containers: Result 574/7/2018 Absolute layout:Example 58 • Suppose that you wanted to arrange a column of buttons (on the left) that take exactly 40% of the width of the container setLayout(null); int width1 = getSize().width * 4 / 10, width2 = getSize().width - width1, height = getSize().height; JPanel buttonPanel = new JPanel(); buttonPanel.setBounds(0, 0, width1, height); buttonPanel.setLayout(new GridLayout(6, 1)); buttonPanel.add(new Label("Buttons", Label.CENTER)); buttonPanel.add(new Button("Button One")); buttonPanel.add(new Button("Button Two")); buttonPanel.add(new Button("Button Three")); buttonPanel.add(new Button("Button Four")); buttonPanel.add(new Button("Button Five")); add(buttonPanel); JPanel everythingElse = new JPanel(); everythingElse.setBounds(width1 + 1, 0, width2, height); everythingElse.add(new Label("Everything Else")); add(everythingElse); 4/7/2018 Turning Off Layout Manager: Result 594/7/2018 Adjusting Space Around Components 60 • Change the space allocated by the layout manager – Most LayoutManagers accept a horizontal spacing (hGap) and vertical spacing (vGap) argument – For GridBagLayout, change the insets • Use a Canvas or a Box as an invisible spacer – For AWT layouts, use a Canvas that does not draw or handle mouse events as an “empty” component for spacing. – For Swing layouts, add a Box as an invisible spacer to improve positioning of components 4/7/2018 Drag-and-Drop Swing GUI Builders 61 • Free: Matisse – Started in NetBeans (now "NetBeans GUI Builder") – Also available in MyEclipse. Not in regular Eclipse. • Commercial – WindowBuilder • instantiations.com. – JFormDesigner • jformdesigner.com – Jvider • jvider.com – SpeedJG • wsoftware.de – Jigloo • 4/7/2018
File đính kèm:
bai_giang_cong_nghe_java_chuong_9_gui_programming_swing_tran.pdf