Bài giảng Phát triển phần mềm nguồn mở - Bài 11: Database, Migrations & Seeding (Tiếp) - Nguyễn Hữu Thể
Giới thiệu
− Laravel kết nối tới các database và thực thi các query
với nhiều database back-ends thông qua sử dụng
• raw SQL,
• fluent query builder,
• Eloquent ORM.
− Hiện tại, Laravel hỗ trợ sẵn 4 database:
• MySQL
• Postgres
• SQLite
• SQL Server
Bạn đang xem 20 trang mẫu của tài liệu "Bài giảng Phát triển phần mềm nguồn mở - Bài 11: Database, Migrations & Seeding (Tiếp) - Nguyễn Hữu Thể", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Phát triển phần mềm nguồn mở - Bài 11: Database, Migrations & Seeding (Tiếp) - Nguyễn Hữu Thể
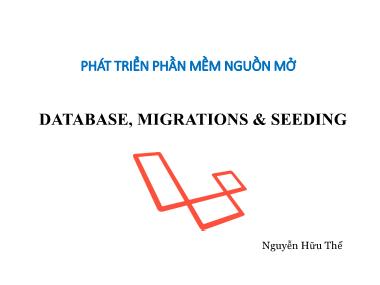
DATABASE, MIGRATIONS & SEEDING Nguyễn Hữu Thể PHÁT TRIỂN PHẦN MỀM NGUỒN MỞ Database ❖ Introduction ❖ Configuration ❖ Read & Write Connections 2 Giới thiệu − Laravel kết nối tới các database và thực thi các query với nhiều database back-ends thông qua sử dụng • raw SQL, • fluent query builder, • Eloquent ORM. − Hiện tại, Laravel hỗ trợ sẵn 4 database: • MySQL • Postgres • SQLite • SQL Server 3 Cấu hình − Thư mục config/database.php. • Trong file này: có thể định nghĩa tất cả các kết nối cơ sở dữ liệu, cũng như chỉ định connection nào là mặc định. ❖ Cấu hình SQL Server 4 'sqlsrv' => [ 'driver' => 'sqlsrv', 'host' => env('DB_HOST', 'localhost'), 'database' => env('DB_DATABASE', 'forge'), 'username' => env('DB_USERNAME', 'forge'), 'password' => env('DB_PASSWORD', ''), 'charset' => 'utf8', 'prefix' => '', ], Đọc & ghi các kết nối 5 'mysql' => [ 'read' => [ 'host' => '192.168.1.1', ], 'write' => [ 'host' => '196.168.1.2' ], 'driver' => 'mysql', 'database' => 'database', 'username' => 'root', 'password' => '', 'charset' => 'utf8', 'collation' => 'utf8_unicode_ci', 'prefix' => '', ], Thiết lập database trong file cấu hình chung .env (Tên_Project/.env) 6 APP_ENV=local APP_KEY=base64:SPqqJfE1ADzonR ot2o5g9J8Ix3iRVHsFOclr0KC1KHI= APP_DEBUG=true APP_LOG_LEVEL=debug APP_URL= DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=ten_database DB_USERNAME=root DB_PASSWORD= BROADCAST_DRIVER=log CACHE_DRIVER=file SESSION_DRIVER=file QUEUE_DRIVER=sync REDIS_HOST=127.0.0.1 REDIS_PASSWORD=null REDIS_PORT=6379 MAIL_DRIVER=smtp MAIL_HOST=mailtrap.io MAIL_PORT=2525 MAIL_USERNAME=null MAIL_PASSWORD=null MAIL_ENCRYPTION=null PUSHER_APP_ID= PUSHER_KEY= PUSHER_SECRET= Thực thi lệnh select 7 namespace App\Http\Controllers; use Illuminate\Support\Facades\DB; use App\Http\Controllers\Controller; class UserController extends Controller { public function index() { $users = DB::select('select * from users where active = ?', [1]); return view('user.index', ['users' => $users]); } } Có thể thực thi câu query sử dụng liên kết đặt tên: $results = DB::select('select * from users where id = :id', ['id' => 1]); Thực thi lệnh select 8 Syntax array select(string $query, array $bindings = array()) Parameters •$query(string) – query to execute in database •$bindings(array) – values to bind with queries Returns array Description Run a select statement against the database. Thực thi câu lệnh insert − Hàm insert nhận câu raw SQL query ở tham số đầu tiên, và bindings ở tham số thứ hai 9 DB::insert('insert into users (id, name) values (?, ?)', [1, ‘Tom']); Syntax bool insert(string $query, array $bindings = array()) Parameters •$query(string) – query to execute in database •$bindings(array) – values to bind with queries Returns bool Description Run an insert statement against the database. Thực thi câu lệnh update − Hàm update: update các records đang có trong cơ sở dữ liệu. Số lượng row ảnh hưởng bởi câu lệnh sẽ được trả về qua hàm này 10 $affected = DB::update('update users set votes = 100 where name = ?', ['John']); Syntax int update(string $query, array $bindings = array()) Parameters •$query(string) – query to execute in database •$bindings(array) – values to bind with queries Returns int Description Run an update statement against the database. Thực thi câu lệnh delete − Hàm delete: xoá các records khỏi cơ sở dữ liệu. Giống như update, số lượng dòng bị xoá sẽ được trả về 11 $deleted = DB::delete('delete from users'); Syntax int delete(string $query, array $bindings = array()) Parameters •$query(string) – query to execute in database •$bindings(array) – values to bind with queries Returns int Description Run a delete statement against the database. Thực thi một câu lệnh chung − Một vài câu lệnh cơ sở dữ liệu không trả về giá trị gì cả. Với những thao tác kiểu này, có thể sử dụng hàm statement trong DB facade 12 DB::statement('drop table users'); Database Example − Table student − We will see how to add, delete, update and retrieve records from database using Laravel in student table. 13 Column Name Column Datatype Extra Id int(11) Primary key | Auto increment Name varchar(25) Database Example - insert − Step 1 − Execute the below command to create a controller called StudInsertController php artisan make:controller StudInsertController 14 15 namespace App\Http\Controllers; use Illuminate\Http\Request; use DB; use App\Http\Requests; use App\Http\Controllers\Controller; class StudInsertController extends Controller { public function insertform(){ return view('stud_create'); } public function insert(Request $request){ $name = $request->input('stud_name'); DB::insert('insert into student (name) values(?)',[$name]); echo "Record inserted successfully."; echo 'Click Here to go back.'; } } Step 2 − Code file app/Http/Controllers/StudInsertController.php 16 Step 3 − Create a view file resources/views/stud_create.php Student Management | Add "> Name 17 Step 4 − Add the following lines in routes\web.php Route::get('insert','StudInsertController@insertform'); Route::post('create','StudInsertController@insert'); Step 5 − Visit the following URL to insert record in database. Database Example - Update − Step 1 − Execute the below command to create a controller called StudViewController. php artisan make:controller StudUpdateController 18 namespace App\Http\Controllers; use Illuminate\Http\Request; use DB; use App\Http\Requests; use App\Http\Controllers\Controller; class StudUpdateController extends Controller { public function index(){ $users = DB::select('select * from student'); return view('stud_edit_view',['users'=>$users]); } public function show($id) { $users = DB::select('select * from student where id = ?',[$id]); return view('stud_update',['users'=>$users]); } public function edit(Request $request,$id) { $name = $request->input('stud_name'); DB::update('update student set name = ? where id = ?',[$name,$id]); echo "Record updated successfully."; echo 'Click Here to go back.'; } } Step 2 − Code file app/Http/Controllers/ StudUpdateController.php Step 3 − Create a view resources/views/stud_edit_view.blade.php View Student Records ID Name Edit @foreach ($users as $user) {{ $user->id }} {{ $user->name }} id }}'>Edit @endforeach Step 4 − Create another view resources/views/stud_update.php Student Management | Edit id; ?>" method = "post"> "> Name<input type = 'text' name = 'stud_name' value = 'name; ?>'/> Step 5 − Add the following lines in routes\web.php Route::get('edit-records','StudUpdateController@index'); Route::get('edit/{id}','StudUpdateController@show'); Route::post('edit/{id}','StudUpdateController@edit'); Step 6 − Visit the following URL to update records in database. Database Example - Delete − Step 1 − Execute the below command to create a controller called StudDeleteController. php artisan make:controller StudDeleteController Database Example - Delete − Step 2 − Code file app/Http/Controllers/StudDeleteController.php namespace App\Http\Controllers; use Illuminate\Http\Request; use DB; use App\Http\Requests; use App\Http\Controllers\Controller; class StudDeleteController extends Controller { public function index(){ $users = DB::select('select * from student'); return view('stud_delete_view',['users'=>$users]); } public function destroy($id) { DB::delete('delete from student where id = ?',[$id]); echo "Record deleted successfully."; echo 'Click Here to go back.'; } } Step 3 − Create a view resources/views/stud_delete_view.blade.php View Student Records IDNameEdit @foreach ($users as $user) {{ $user->id }} {{ $user->name }} id }}'>Delete @endforeach Step 4 − Add the following lines in routes/web.php Route::get('delete-records','StudDeleteController@index'); Route::get('delete/{id}','StudDeleteController@destroy'); Schema Builder 28 − Là một class trong bộ Facades của Laravel, nó giúp chúng ta làm việc với tất cả các cơ sở dữ liệu mà Laravel hỗ trợ với các hàm được định nghĩa sẵn. − Kết hợp với Migrations để xây dự cấu trúc database. − Namespace của Schema Builder: Illuminate\Support\Facades\Schema Schema Builder – Tạo table 29 Vd: Tạo bảng users. Schema::table('users', function ($table) { //code }); Schema::table('tablename', function ($table) { //code }); Schema – Khóa chính, Khóa ngoại 30 Tạo khóa chính: $table->primary(‘TenKhoaChinh’); Tạo khóa chính $table->String(‘TenKhoaChinh’,10)->primary(); Khóa chính kiểu chuỗi $table->increments(TenKhoaChinh’); Khóa chính kiểu số nguyên tự động tăng Tạo Khóa ngoại: $table->foreign(‘KhoaNgoai’)-> references(‘KhoaChinh’)->on(‘Bang’); Schema – Kiểu dữ liệu 31 Command Description $table->bigIncrements('id'); Tăng ID (primary key) "UNSIGNED BIG INTEGER". $table->bigInteger('votes'); Tương đương với BIGINT. $table->boolean('confirmed'); Tương đương với BOOLEAN. $table->char('name', 4); Tương đương với CHAR với độ dài cho trước. $table->date('created_at'); Tương đương với DATE. $table->dateTime('created_at'); Tương đương với DATETIME. $table->dateTimeTz('created_at'); Tương đương với DATETIME (with timezone). $table->decimal('amount', 5, 2); Tương đương với DECIMAL với độ chính sách và phần thập phân. $table->double('column', 15, 8); Tương đương với DOUBLE với độ chính xác, 15 chữ số và 8 ký tự tính sau dấu phảy. $table->float('amount', 8, 2); Tương đương với FLOAT, 8 chữ số and 2 chữ số tính sau dấu phẩy. $table->increments('id'); Tăng ID (primary key) "UNSIGNED INTEGER". $table->integer('votes'); Tương đương với INTEGER. Schema – Kiểu dữ liệu 32 Command Description $table->longText('description'); Tương đương với LONGTEXT. $table->mediumIncrements('id'); Tăng ID (primary key) UNSIGNED MEDIUM INTEGER". $table->mediumInteger('numbers'); Tương đương với MEDIUMINT. $table->mediumText('description'); Tương đương với MEDIUMTEXT. $table->rememberToken(); Thêm remember_token như VARCHAR(100) NULL. $table->smallIncrements('id'); Tăng ID (primary key) "UNSIGNED SMALL INTEGER". $table->smallInteger('votes'); Tương đương với SMALLINT. $table->string('email'); Tương đương với VARCHAR . $table->string('name', 100); Tương đương với VARCHAR với độ dài. $table->text('description'); Tương đương với TEXT. $table->time('sunrise'); Tương đương với TIME. $table->timeTz('sunrise'); Tương đương với TIME (với timezone). $table->tinyInteger('numbers'); Tương đương với TINYINT. $table->timestamp('added_on'); Tương đương với TIMESTAMP. $table->timestampTz('added_on'); Tương đương với TIMESTAMP (với timezone). Schema – Kiểu dữ liệu 33 Command Description $table->unsignedBigInteger('votes'); Tương đương với Unsigned BIGINT. $table->unsignedInteger('votes'); Tương đương với Unsigned INT. $table->unsignedMediumInteger('votes'); Unsigned MEDIUMINT. $table->unsignedSmallInteger('votes'); Unsigned SMALLINT. $table->unsignedTinyInteger('votes'); Tương đương với Unsigned TINYINT. $table->uuid('id'); Tương đương với UUID. Schema – Kiểu dữ liệu 34 Modifier Description ->after('column') Đặt column "after" một column khác (MySQL Only) ->comment('my comment') Thêm một comment cho column. ->default($value) Đặt giá trị "mặc định" vào column ->first() Đặt column "first" vào trong bảng (MySQL Only) ->nullable() Cho phép dữ liệu kiểu NULL có thể chèn vào column. ->storedAs($expression) Tạo một cột stored (MySQL Only) ->unsigned() Đặt cột integer sang UNSIGNED ->virtualAs($expression) Tạo một cột virtual (MySQL Only) Schema – Tạo table − Tạo table trong route 35 Route::get('database', function (){ Schema::create('loaisanpham', function($table){ $table->increments('id'); $table->string('ten', 200); //kiểu varchar }); echo "Đã tạo bảng loaisanpham"; }); Ví dụ: tạo table với giá trị mặc định hoặc null 36 Schema::create('theloai', function($table){ $table->increments('id'); $table->string('ten', 200)->nullable(); //kiểu varchar $table->string('nsx')->default('Samsung'); }); echo "Đã tạo bảng"; Liên kết khóa chính, khóa ngoại 37 Route::get ( 'lienketbang', function () { Schema::create ( 'sanpham', function ($table) { $table->increments ( 'id' ); $table->string ( 'ten' ); // kiểu varchar, mặc định 255 $table->float ( 'gia' ); $table->integer('soluong')->default(0); $table->integer('id_loaisanpham')->unsigned(); $table->foreign('id_loaisanpham')->references('id')- >on('loaisanpham'); } ); echo "Đã tạo bảng liên kết"; } ); Sửa table 38 $table->dropColumn('TenCot'); Xóa cột trong bảng Schema::rename($from, $to); Đổi tên bảng Route::get ( 'suabang', function () { Schema::table ( 'theloai', function ($table) { $table->dropColumn('nsx'); } ); } ); Route::get ( 'themcot', function () { Schema::table ( 'theloai', function ($table) { $table->string( 'email' ); } ); } ); Route::get ( 'doiten', function () { Schema::rename ( 'theloai', 'nguoidung'); } ); Xóa table 39 Schema::drop('users'); Xóa bảng users Schema::dropIfExists('users'); Xóa bảng users nếu bảng tồn tại Route::get ( 'xoabang', function () { Schema::drop ( 'nguoidung' ); } ); Kiểm tra nếu bảng tồn tại thì mới xóa Route::get ( 'xoabang', function () { Schema::dropIfExist ( 'nguoidung' ); } ); Migrations ❖ Introduction ❖ Generating Migrations ❖ Migration Structure ❖ Running Migrations ❖ Rolling Back Migrations 40 Migrations − Migration được coi như là version control cho database, cho phép team có thể dễ dàng thay đổi và chia sẻ schema của database trong chương trình với nhau. − Migration cơ bản được sử dụng cùng với schema builder để dễ dàng xây dựng cấu trúc cho database schema. Nếu chúng ta đã gặp vấn đề khi thêm một cột vào local của các đồng đội trong team, migration sẽ xử lý vấn đề này rất dễ dàng. − Schema facade hỗ trợ việc tạo và thao tác trên các bảng mà không cần biết về database, tương ứng và mạch lạc khi giao tiếp với các hệ thống database khác nhau mà Laravel hỗ trợ. 41 Tạo Migrations − File migration mới sẽ được đặt trong thư mục database/migrations. Mỗi file migration được đặt tên bao gồm timestamp để xác định thứ tự các migration với nhau. − Tham số --create=TenBang Migrate tạo bảng --table=TenBang Migrate chỉnh sửa bảng 42 php artisan make:migration TenMigrate php artisan make:migration create_users_table php artisan make:migration create_users_table --create=users php artisan make:migration add_votes_to_users_table --table=users Cấu trúc migration 43 use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateFlightsTable extends Migration { public function up() //đoạn lệnh khi thực hiện migrate { Schema::create('flights', function (Blueprint $table) { $table->increments('id'); $table->string('name'); $table->string('airline'); $table->timestamps(); }); } public function down() //đoạn lệnh thực hiện khi Rollback { Schema::drop('flights'); } } Thực thi Migrations − Để thực thi tất cả các migration trong chương trình, sử dụng lệnh: 44 php artisan migrate Rolling Back Migrations − Lệnh migrate:reset sẽ thực hiện rollback lại toàn bộ migration của chương trình − Rollback & Migrate trong một câu lệnh • Lệnh migrate:refresh sẽ rollback lại toàn bộ migration của chương trình, và thực hiện câu lệnh migrate. Câu lệnh sẽ thực hiện tái cấu trúc toàn bộ database: 45 php artisan migrate:refresh php artisan migrate:rollback php artisan migrate:reset - Hủy bỏ việc thực thi của migrate trước Ví dụ demo: tạo tên database laravelk (trống, chưa có table) − Vào thư mục project: Nhấn Ctrl+Shift+ Chuột phải > Open Command Promt Here − Gõ vào CMD: − Xuất hiện 3 table: 46 C:\xampp\htdocs\laravelk>php artisan migrate Migration table created successfully. Migrated: 2014_10_12_000000_create_users_table Migrated: 2014_10_12_100000_create_password_resets_table Bảng lưu lại quá trình tạo bảng − batch = 1: mới tạo lần đầu 47 Tạo migration table_SanPham 48 C:\xampp\htdocs\laravelk>php artisan make:migration table_SanPham Created Migration: 2016_12_19_130901_table_SanPham Kiểm tra: database\migrations\2016_12_19_130901_table_SanPham.php 49 use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class TableSanPham extends Migration{ //Viết code cho migration public function up() { Schema::create('sanpham', function($table){ $table->increments('id'); $table->string('ten'); $table->integer('soluong'); }); } public function down() { Schema::drop('sanpham'); } } Run Migrate 50 C:\xampp\htdocs\laravelk>php artisan migrate Migrated: 2016_12_19_130901_table_SanPham Kiểm tra table Kiểm tra migrate Hủy migrate, quay lại phiên trước php artisan migrate:rollback 51 C:\xampp\htdocs\laravelk>php artisan migrate:rollback Rolled back: 2016_12_19_130901_table_SanPham Kiểm tra: đã xóa mất table_SanPham Hủy bỏ hết công việc của migrate php artisan migrate:reset 52 Kiểm tra: đã mất tất cả các table do migration tạo ra. C:\xampp\htdocs\laravelk>php artisan migrate:reset Rolled back: 2014_10_12_100000_create_password_resets_table Rolled back: 2014_10_12_000000_create_users_table Seeding ❖ Introduction ❖ Writing Seeders ❖ Running Seeders 53 Seeding − Laravel có một phương thức đơn giản để seed database với dữ liệu test sử dụng các seed class. − Tất cả các seed class được lưu trong thư mục database/seeds. − Viết Seeders • Để sinh ra một seeder, gọi lệnh make:seeder Artisan. Tất cả các seeder được sinh ra bởi framework sẽ được đặt trong thư mục database/seeds: 54 php artisan make:seeder UsersTableSeeder Seeding 55 use Illuminate\Database\Seeder; use Illuminate\Database\Eloquent\Model; class DatabaseSeeder extends Seeder { public function run() //Viết code tạo table users { DB::table('users')->insert([ 'name' => str_random(10), 'email' => str_random(10).'@gmail.com', 'password' => bcrypt('secret'), ]); } } Thực thi Seeders − Khi đã có seeder class • Có thể sử dụng câu lệnh db:seed Artisan để seed vào database. • Mặc định, câu lệnh db:seed thực thi class DatabaseSeeder, mà các bạn có thể sử dụng để gọi các seed class khác. • Tuy nhiên, bạn cũng có thể sử dụng tuỳ chọn --class để chỉ định thực hiện một seed class nào đó. php artisan db:seed php artisan db:seed --class=UsersTableSeeder 56 Ví dụ: tạo table users 57 use Illuminate\Database\Seeder; class DatabaseSeeder extends Seeder { public function run() { DB::table ( 'users' )->insert ( [ 'name' => 'Tom', 'email' => 'tom@gmail.com', 'password' => bcrypt('123456') ] ); } } Mở cửa sổ cmd : php artisan db:seed Kiểm tra dữ liệu:
File đính kèm:
bai_giang_phat_trien_phan_mem_nguon_mo_bai_11_database_migra.pdf