Bài giảng Công nghệ Java - Chương 11: Basic Swing Component - Trần Quang Diệu
Review
The Java Foundation Classes (JFC) are developed as an
extension to Abstract Windows Toolkit (AWT), to
overcome the shortcomings of AWT.
Swing is a set of classes under the JFC that provide
lightweight visual components and enable creation of
an attractive GUI.
Swing Applets provide support for assistive
technologies and a RootPane (and thus support for
adding menu bar).
With Swing, most of the components can display text
as well as images.
Bạn đang xem 20 trang mẫu của tài liệu "Bài giảng Công nghệ Java - Chương 11: Basic Swing Component - Trần Quang Diệu", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Công nghệ Java - Chương 11: Basic Swing Component - Trần Quang Diệu
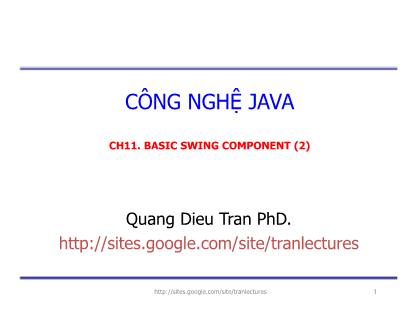
Swing - II CÔNG NGHỆ JAVA CH11. BASIC SWING COMPONENT (2) Quang Dieu Tran PhD. 1 2Review The Java Foundation Classes (JFC) are developed as an extension to Abstract Windows Toolkit (AWT), to overcome the shortcomings of AWT. Swing is a set of classes under the JFC that provide lightweight visual components and enable creation of an attractive GUI. Swing Applets provide support for assistive technologies and a RootPane (and thus support for adding menu bar). With Swing, most of the components can display text as well as images. 3Objectives Describe menus Discuss trees and tables Discuss progress bars Discribe Dialog box Discuss JSpinner Discuss MVC architecture Discuss features of Swing such as: KeyStroke handling, Action Objects, Nested Containers Virtual desktops, compound borders Drag and Drop Java 2D Customized Dialogs, Standard Dialog classes 4Menus Menus show a list of items that indicate various tasks. Menus display several options that are broadly categorized. Select or click an option and another list or sub-menu opens up. A Swing menu consists of a menubar, menu_items and menus. Menubar is the root of all menus and menu items. 5Menus components 6Hierarchy JMenuBar JPopupMenu JAbstractButton JSeperator JMenuItem JMenu JCheckBoxMenuItem JRadioButtonMenuItem JComponent Container Component Object 7JMenuBar JMenuBar is a component that can be added to a container through a JFrame, JWindow or JInternalFrame as its rootpane. Consists of a number of JMenus with each JMenu represented as a string within the JMenuBar JMenuBar requires two additional classes to supplement its work. They are: SingleSelectionModel class – keeps track of the currently selected menu LookAndFeel class – Responsible for drawing the menu bar and responding to events that occur in it 8JMenu JMenu is seen as a text string under JMenuBar and it acts as a popup menu when the user clicks on it. JMenu can have standard menu items such as JMenuItem, JCheckBoxMenuItem, JRadioButtonMenuItem as well as JSeparator. JMenu has two additional classes: JPopupMenu – Used to display the JMenu’s menu items when the user clicks on a JMenu. LookAndFeel – Responsible for drawing the menu in the menubar and for responding to all events that occur in it. 9JPopupMenu Displays an expanded form of menu. Used for the “pull-right” menu. It is also used as a shortcut menu, which is activated by the right click of the mouse. The constructors used to create JPopupMenu are: public JPopupMenu() – creates a JPopupMenu. public JPopupMenu(String label) – creates a JPopupMenu with the specified title. 10 Functions of JPopupMenu Method Purpose public JMenuItem add(JMenuItem menuItem) Appends the specified menu item at the end of the menu public JMenuItem add(String s) Creates a new menu item with the specified text and appends it to the end of the menu public void show(Component c, int x, int y) Displays the popup menu at the position (x,y) in the coordinate space of the component "c" public boolean isPopupTrigger() Determines whether the mouse event is considered as the popup trigger for the current platform 11 JMenuItem It is a component that appears as a text string possibly with an icon in a JMenu or JPopupMenu. A dialog box is displayed when the user selects a JMenuItem that is followed by ellipses. Dialog Box JMenuItem dissappears 12 JCheckBoxMenuItem It is a sub class of the JMenuItem class. Contains checkboxes as its items. Checkboxes are created using JCheckBox class. Information about external state of checkbox item such as text string , an icon and background colour can be changed. When a JCheckBoxMenuItem is clicked and released, the state of a menu item changes to selected or deselected. 13 JRadioButtonMenuItem Similar to checkboxes except that only one radio button can be selected at any point of time. May have a text string and/or an icon. The two possibilities that may happen when a radio button is selected are: Clicking a selected radio button does not change its state. Clicking an unselected radio button deselects the earlier selected one and selects the current button. 14 public class MenuTest extends JFrame { public MenuTest(String title) { super(title); JMenuBar mb = new JMenuBar(); JMenu fileMenu = new JMenu("Display"); JMenu pullRightMenu = new JMenu("Pull right"); fileMenu.add("Welcome"); fileMenu.addSeparator(); fileMenu.add(pullRightMenu); fileMenu.add("Exit"); pullRightMenu.add(new JCheckBoxMenuItem("Good morning!")); pullRightMenu.add(new JCheckBoxMenuItem("Good afternoon!")); pullRightMenu.add(new JCheckBoxMenuItem("Good night! Ciao!")); mb.add(fileMenu); setJMenuBar(mb); setSize(400, 300); setVisible(true); } public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { new MenuTest("Menu Test"); } }); } } Example Output 15 JPopupMenu – Example class PopupMenuTest extends JPanel { JLabel lbl; JPopupMenu popupMenu; public PopupMenuTest() { lbl = new JLabel("Click the right mouse button"); popupMenu = new JPopupMenu(); JMenuItem menu1 = new JMenuItem("Orange"); JMenuItem menu2 = new JMenuItem("Pineapple"); JMenuItem menu3 = new JMenuItem("Mango"); menu1.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { lbl.setText("Orange"); } }); menu2.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { lbl.setText("Pineapple"); } }); 16 JPopupMenu – Example Contd menu3.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { lbl.setText("Mango"); } }); popupMenu.add(menu1); popupMenu.add(menu2); popupMenu.add(menu3); addMouseListener(new MouseAdapter() { public void mouseReleased(MouseEvent e) { if (e.isPopupTrigger()) { popupMenu.show(e.getComponent(), e.getX(), e.getY()); } } }); add(lbl); } public static void main(String args[]) { EventQueue.invokeLater(new Runnable() { public void run() { JFrame frame = new JFrame("Popup Menu"); PopupMenuTest p1 = new PopupMenuTest(); frame.getContentPane().add("Center", p1); frame.getContentPane().setBackground(Color.GRAY); frame.setSize(175, 175); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }); } } JPopupMenu – Example Contd Output 17 18 public class MenuItems extends JApplet { public void init() { Icon newIcon = new ImageIcon("new.gif", "Create a new document"); Icon openIcon = new ImageIcon("open.gif", "Open an existing document"); JMenuBar mb = new JMenuBar(); JMenu fileMenu = new JMenu("File"); JMenuItem newItem = new JMenuItem("New", newIcon); JMenuItem openItem = new JMenuItem("Open ...", openIcon); JMenuItem saveItem = new JMenuItem("Save"); JMenuItem saveAsItem = new JMenuItem("Save As ..."); JMenuItem exitItem = new JMenuItem("Exit", 'x'); JMenuItem - Example fileMenu.add(newItem); fileMenu.add(openItem); fileMenu.add(saveItem); fileMenu.add(saveAsItem); fileMenu.addSeparator(); fileMenu.add(exitItem); mb.add(fileMenu); setJMenuBar(mb); } } /* */ Output 19 class CheckMenuTest extends JFrame { JButton b1, b2; JPopupMenu popupMenu; public CheckMenuTest() { super("CheckBox Menu Items"); JPanel p = (JPanel) getContentPane(); p.setLayout(new BoxLayout(p, BoxLayout.Y_AXIS)); b1 = new JButton("Click here"); b2 = new JButton("Exit"); p.add(b1); p.add(b2); popupMenu = new JPopupMenu(); JMenuItem menu1 = new JMenuItem("JMenuItem"); popupMenu.add(menu1); JCheckBoxMenuItem jcb1 = new CheckBoxMenuItem("Item1"); JCheckBoxMenuItem jcb2 = new CheckBoxMenuItem("Item2"); JCheckBoxMenuItem jcb3 = new CheckBoxMenuItem("Item3"); popupMenu.add(jcb1); popupMenu.add(jcb2); popupMenu.add(jcb3); popupMenu.addSeparator(); PopupMenu - Example 20 JRadioButtonMenuItem jrb1 = new JRadioButtonMenuItem("JRadioButtonMenuItem1"); JRadioButtonMenuItem jrb2 = new JRadioButtonMenuItem("JRadioButtonMenuItem2"); jrb1.setSelected(true); ButtonGroup bg = new ButtonGroup(); bg.add(jrb1); bg.add(jrb2); popupMenu.add(jrb1); popupMenu.add(jrb2); b1.addMouseListener(new myListener()); } class myListener extends MouseAdapter { public void mouseReleased(MouseEvent e) { popupMenu.show((JComponent) e.getSource(), e.getX(), e.getY()); } } PopupMenu - Example 21 PopupMenu – Example Contd public static void main(String args[]) { CheckMenuTest p1 = new CheckMenuTest(); p1.setForeground(Color.black); p1.setBackground(Color.lightGray); p1.setSize(300,200); p1.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); p1.setVisible(true); } } Output 22 Trees A Tree depicts information in a hierarchical, vertical manner. Windows Explorer has a tree like structure depicting files and folders. Windows Explorer structures can be created in Java using JTree. Every row in the hierarchy is termed as a node. By default, the tree displays the root node. A node having child nodes is called a branch node else it is called as a leaf node. 23 Example public class TreeDemo extends JFrame { public static void main(String args[]) { TreeDemo frame = new TreeDemo("Tree Demo"); frame.setSize(300, 200); frame.setVisible(true); } public TreeDemo(String title) { setTitle(title); DefaultMutableTreeNode root = new DefaultMutableTreeNode("Painting"); // create child node DefaultMutableTreeNode style = new DefaultMutableTreeNode("Impressionism"); root.add(style); // create further child nodes DefaultMutableTreeNode artist = new DefaultMutableTreeNode("Caravaggio"); style.add(artist); 24 artist = new DefaultMutableTreeNode("Degas"); style.add(artist); artist = new DefaultMutableTreeNode("Monet"); style.add(artist); // create another child node style = new DefaultMutableTreeNode("Expressionism"); root.add(style); artist = new DefaultMutableTreeNode("Henry Moore"); style.add(artist); artist = new DefaultMutableTreeNode("Salvador Dali"); style.add(artist); JTree jt = new JTree(root); Container contentPane = getContentPane(); contentPane.add(new JScrollPane(jt)); } } Example Contd Output When the nodes are clicked 25 Tables Volumes of data are better maintained in a tabular format than as a list. Useful in storing numerical data. Certain computer applications require tables to display data allowing the user to edit them. JTable class in Swing enables us to create tables. JTable does not store data, it only provides a view of it. 26 class StudentFrame extends JFrame { public static void main(String args[]) { StudentFrame sf = new StudentFrame(); sf.setSize(200, 200); sf.setVisible(true); } public StudentFrame() { Object[][] cells = { { "ACJTP", new Integer(01), new Integer(40000) }, { "ACEP", new Integer(02), new Integer(50000) }, { "eACCP", new Integer(03), new Integer(70000) }, }; String[] colnames = { "Coursename", "Coursecode", "Fees" }; JTable table = new JTable(cells, colnames); Container contentPane = getContentPane(); contentPane.add(new JScrollPane(table), "Center"); } } Example Output 27 Progress Bars Monitoring the progress of any activity can be done by using percentage figures. A better way to do the same is by using charts or bar graphs. Certain programs provide a graphical indication of a task that is currently under progress. Swing uses JProgressBar class to implement the same. 28 Example class ProgressBarDemo extends JFrame implements ActionListener { JLabel l = new JLabel("Enter a score out of 1000"); JProgressBar pbar1; JProgressBar pbar2; JButton done; JTextField tf; Container contentPane; public static final int MAXSCORE = 1000; public ProgressBarDemo() { super("ProgressBarDemo"); done = new JButton("Done"); done.addActionListener(this); pbar1 = new JProgressBar(0, MAXSCORE); pbar1.setStringPainted(true); pbar1.setValue(MAXSCORE); tf = new JTextField(10); contentPane = getContentPane(); contentPane.setLayout(new FlowLayout()); 29 Example Contd contentPane.add(l); contentPane.add(tf); contentPane.add(done); contentPane.add(pbar1); } public void actionPerformed(ActionEvent e) { if (e.getSource().equals(done)) { String score = tf.getText(); int scoreval = Integer.parseInt(score); pbar2 = new JProgressBar(0, MAXSCORE); pbar2.setValue(scoreval); pbar2.setStringPainted(true); contentPane.add(pbar2); validate(); } } Output 30 JSpinner It is a single line input field that lets the user select a number or an object value from an ordered set . JSpinner has an editor that displays the values and buttons on the right hand side to move through the values. JSpinner is used for entering a value. SpinnerModel is used to represent a sequence of values. SpinnerListModel constructs a SpinnerModel with a sequence of elements given in the list or array. The editor will monitor the model with a ChangeListener and will display the current value returned by SpinnerModel.getValue() method. 31 Example public class SpinnerDemo extends JPanel { public SpinnerDemo(boolean cycleMonths) { super(new SpringLayout()); String labels = "Month: "; Calendar calendar = Calendar.getInstance(); JFormattedTextField ftf = null; String[] monthStrings = getMonthStrings(); SpinnerListModel monthModel = null; monthModel = new SpinnerListModel(monthStrings); JSpinner spinner = addLabeledSpinner(this, labels, monthModel); ftf = getTextField(spinner); if (ftf != null) { ftf.setColumns(8); // specify more width than we need ftf.setHorizontalAlignment(JTextField.RIGHT); } } 32 public JFormattedTextField getTextField(JSpinner spinner) { JComponent editor = spinner.getEditor(); if (editor instanceof JSpinner.DefaultEditor) { return ((JSpinner.DefaultEditor) editor).getTextField(); } else { System.err.println("Different editor : " + spinner.getEditor().getClass() + " isn't a descendant of DefaultEditor"); return null; } } static protected String[] getMonthStrings() { String[] months = new DateFormatSymbols().getMonths(); int lastIndex = months.length - 1; if (months[lastIndex] == null || months[lastIndex].length() <= 0) { // last item empty String[] monthStrings = new String[lastIndex]; System.arraycopy(months, 0, monthStrings, 0, lastIndex); return monthStrings; } Example 33 else { // last item not empty return months; } } static protected JSpinner addLabeledSpinner(Container c, String label, SpinnerModel model) { JLabel l = new JLabel(label); c.add(l); JSpinner spinner = new JSpinner(model); l.setLabelFor(spinner); c.add(spinner); return spinner; } public static void main(String[] args) { JFrame frame = new JFrame("Spinner Demo"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JComponent newContentPane = new SpinnerDemo(false); frame.setContentPane(newContentPane); frame.setVisible(true); frame.setSize(100, 50); } } Example Contd Output 34 Dialog Box JOptionPane provides modal dialog box. To create a non modal dialog box the dialog box should extend from JDialog class. JOptionPanes’s showXXXXDialog methods are more commonly used to display a modal dialog box. The two most useful showXxxxDialog methods are showMessageDialog and showOptionDialog. showMessageDialog displays only one button . showOptionDialog method displays a customized dialog which can display a variety of buttons with customized text written on it and can contain standard text messages or a collection of components. 35 Example import javax.swing.*; import java.awt.*; import java.awt.event.*; import javax.swing.BorderFactory; import javax.swing.border.Border; class dialogDemo extends JPanel { JLabel label; JFrame frame; String simpleDialogDesc = "Some simple message dialogs"; String moreDialogDesc = "Some complex message dialogs"; public dialogDemo(JFrame frame) { super(new BorderLayout()); this.frame = frame; // creating two panels to be used in tabbed panes JPanel frequentPanel = createSimpleBox(); JPanel featurePanel = createComplexFeatureBox(); label = new JLabel("Click the \"Show it!\" button" +" to show the different dialog boxes.",JLabel.CENTER); Border padding = BorderFactory.createEmptyBorder(20,20,5,20); frequentPanel.setBorder(padding); featurePanel.setBorder(padding); //creating the tabbed panes JTabbedPane tPane = new JTabbedPane(); //adding to the first tabbed pane the features of SimpleDialog tPane.addTab("Simple Modal Dialogs" , null,frequentPanel,simpleDialogDesc); //adding to the second tabbed pane the features of complex //Dialog box tPane.addTab("More Complex Dialogs", null,featurePanel, moreDialogDesc); add(tPane, BorderLayout.CENTER); add(label, BorderLayout.PAGE_END); label.setBorder(BorderFactory.createEmptyBorder(10,10,10,10)); } /** Sets the text displayed at the bottom of the frame. */ void setLabel(String newText) { label.setText(newText); } /** Creates the panel shown by the first tab. */ private JPanel createSimpleBox() { final int numButtons = 4; JRadioButton[] radioButtons = new JRadioButton[numButtons]; final ButtonGroup group = new ButtonGroup(); JButton showItButton = null; 36 Example Contd final String defaultMessageCommand = "default"; final String ynCommand = "yesno"; final String yeahNahCommand = "yeahnah"; final String yncCommand = "ync"; radioButtons[0] = new JRadioButton("OK (in the novices words)"); radioButtons[0].setActionCommand(defaultMessageCommand); radioButtons[1]=new JRadioButton("Yes/No(in the novices words)"); radioButtons[1].setActionCommand(ynCommand); radioButtons[2] = new JRadioButton("Yes/No "+ "(in the programmer's words)"); radioButtons[2].setActionCommand(yeahNahCommand); radioButtons[3] = new JRadioButton("Yes/No/Cancel "+ "(in the programmer's words)"); radioButtons[3].setActionCommand(yncCommand); for (int i = 0; i < numButtons; i++) { group.add(radioButtons[i]); } radioButtons[0].setSelected(true); showItButton = new JButton("Show it!"); showItButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String command = group.getSelection().getActionCommand(); //ok dialog with one button if (command == defaultMessageCommand) { JOptionPane.showMessageDialog(frame,"Trees are supposed to be green."); } //yes/no dialog with two buttons else if (command == ynCommand) { int n = JOptionPane.showConfirmDialog(frame, "Would you like green trees and eggs?","An Absurd Question",JOptionPane.YES_NO_OPTION); if (n == JOptionPane.YES_OPTION) { setLabel("Oops!"); } else if (n == JOptionPane.NO_OPTION) { setLabel("Me neither!"); } else { setLabel("Come on -- tell me!"); } //yes/no (not in those words) } else if (command == yeahNahCommand) { Object[] options = {"Yes, please", "No thanks!"}; 37 Example Contd int n = JOptionPane.showOptionDialog(frame,"Would you like green trees and eggs?","A Silly Question",JOptionPane.YES_NO_OPTION,JOptionPane.QUESTION_MESSAGE, null, options,options[0]); if (n == JOptionPane.YES_OPTION) { setLabel("You're joking!"); } else if (n == JOptionPane.NO_OPTION) { setLabel("I don't like them, either."); } else { setLabel("Come on -- tell the truth!"); } //yes/no/cancel (not in those words) } else if (command == yncCommand) { Object[] options = {"Yes, please","No, thanks","No eggs, no ham!"}; int n = JOptionPane.showOptionDialog(frame,"Would you like some green eggs to go "+ "with that egg?","A Silly Question",JOptionPane.YES_NO_CANCEL_OPTION, JOptionPane.QUESTION_MESSAGE,null,options,options[2]); if (n == JOptionPane.YES_OPTION) { setLabel("Here you go: green eggs and trees!"); } 38 Example Contd else if (n == JOptionPane.NO_OPTION) { setLabel("OK, just the ham, then."); } else if (n == JOptionPane.CANCEL_OPTION) { setLabel("Well, I'm certainly not going to eat them!"); } else { setLabel("Please tell me what you want!"); } } return; } }); return createPane(simpleDialogDesc + ":",radioButtons,showItButton); } private JPanel createPane(String description,JRadioButton[] radioButtons,JButton showButton) { int numChoices = radioButtons.length; JPanel box = new JPanel(); JLabel label = new JLabel(description); box.setLayout(new BoxLayout(box, BoxLayout.PAGE_AXIS)); box.add(label); for (int i = 0; i < numChoices; i++) { box.add(radioButtons[i]); } JPanel pane = new JPanel(new BorderLayout()); pane.add(box, BorderLayout.PAGE_START); pane.add(showButton, BorderLayout.PAGE_END); return pane; } /** Creates the panel shown by the second tab. */ private JPanel createComplexFeatureBox() { final int numButtons = 5; JRadioButton[] radioButtons = new JRadioButton[numButtons]; final ButtonGroup group = new ButtonGroup(); JButton showItButton = null; final String pickOneCommand = "pickone"; final String textEnteredCommand = "textfield"; final String nonAutoCommand = "nonautooption"; final String customOptionCommand = "customoption"; final String nonModalCommand = "nonmodal"; radioButtons[0] = new JRadioButton("Pick one of several choices"); radioButtons[0].setActionCommand(pickOneCommand); radioButtons[1] = new JRadioButton("Enter some text"); radioButtons[1].setActionCommand(textEnteredCommand); 39 Example Contd radioButtons[2] = new JRadioButton("Non-auto-closing dialog"); radioButtons[2].setActionCommand(nonAutoCommand); radioButtons[3] = new JRadioButton("Input-validating dialog " + "(with custom message area)"); radioButtons[3].setActionCommand(customOptionCommand); radioButtons[4] = new JRadioButton("Non-modal dialog"); radioButtons[4].setActionCommand(nonModalCommand); for(int i = 0; i < numButtons; i++) { group.add(radioButtons[i]); } radioButtons[0].setSelected(true); showItButton = new JButton("Show it!"); showItButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String command = group.getSelection().getActionCommand(); //pick one of many if(command == pickOneCommand) { Object[] possibilities = {"ham", "spam", "yam"}; String s = (String)JOptionPane.showInputDialog(frame,"Complete the sentence:\n" + "\"Green eggs and...\"","Customized Dialog",JOptionPane.PLAIN_MESSAGE,null, possibilities,"ham"); //Check whether a string was returned and display it if((s != null) && (s.length() > 0)) { setLabel("Green eggs and... " + s + "!"); return; } //If you're here, the return value was null/empty. setLabel("Come on, finish the sentence!"); //text input } else if (command == textEnteredCommand) { String s = (String)JOptionPane.showInputDialog(frame,"Complete the sentence:\n" +"\"Green eggs and...\"","Customized Dialog",JOptionPane.PLAIN_MESSAGE,null,null,"ham"); //If a string was returned, display it. if ((s != null) && (s.length() > 0)) { setLabel("Green eggs and... " + s + "!"); return; } //If you're here, the return value was null/empty. setLabel("Come on, finish the sentence!"); //non-auto-closing dialog } 40 Example Contd else if (command == nonAutoCommand) { final JOptionPane optionPane = new JOptionPane("The only way to close this dialog is by\n" + "pressing one of the following buttons.\n" + "Do you understand?", JOptionPane.QUESTION_MESSAGE,JOptionPane.YES_NO_OPTION); final JDialog dialog = new JDialog(frame,"Click a button",true); dialog.setContentPane(optionPane); dialog.setDefaultCloseOperation(JDialog.DO_NOTHING_ON_CLOSE); dialog.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent we) { setLabel("Thwarted user attempt to close window."); } }); } else if (command == nonModalCommand) { //Create the non-modal dialog box final JDialog dialog = new JDialog(frame, "A Non-Modal Dialog Box"); JLabel label = new JLabel("" + "This is a non- modal dialog." + "You can have one or more of these " + "and still use the main window."); label.setHorizontalAlignment(JLabel.CENTER); Font font = label.getFont(); label.setFont(label.getFont().deriveFont(font.PLAIN,14.0f)); JButton close = new JButton("Close"); close.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { dialog.setVisible(false); dialog.dispose(); } }); JPanel closePanel = new JPanel(); closePanel.setLayout(new BoxLayout(closePanel,BoxLayout.LINE_AXIS)); closePanel.add(Box.createHorizontalGlue()); closePanel.add(close); closePanel.setBorder(BorderFactory.createEmptyBorder(0,0,5,5)); 41 Example Contd contentPane.add(closePanel, BorderLayout.PAGE_END); dialog.setContentPane(contentPane); //Show it. dialog.setSize(new Dimension(300, 150)); dialog.setLocationRelativeTo(frame); dialog.setVisible(true); } } }); return createPane(moreDialogDesc + ":",radioButtons,showItButton); } public static void main(String [] args) { JFrame frame = new JFrame("Dialog Demo"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); dialogDemo newContentPane = new dialogDemo(frame); frame.setContentPane(newContentPane); frame.setVisible(true); frame.pack(); } } Output 42 MVC Swing helps to specify the look and feel, such as the Java look and feel, Windows look and feel. To achieve this, Swing components work within the MVC model. MVC model stands for: Model View Controller 43 Interaction between MVC Model Controller View View reads the contents Informs view of state change Informs the view to update visuals U p d a te t h e c o n te n ts 44 Characteristics common to Swing Components Content - decides on the state of the component. Visual appearance - determines what the component should look like. Behavior - decides how the component should respond to events. 45 MVC classes Model – Stores the data or the state of the object View – Responsible for showing the component on the basis of the state Controller – Handles the event Interaction between the classes are controlled by the MVC pattern. Each class has necessary methods defined within them. 46 MVC - Example ControllerEvent notification ModelView Calls related methods to make necessary changes Informs about the change of state Redraws the component 47 Swing features KeyStrokeHandling, ActionObjects, Nested Containers Keystrokes can be captured by creating a KeyStroke object and registering it with the component. Action interface extends ActionListener specifying an enabled property as well as properties for text descriptions. Since the fundamental class, JComponent, contains a RootPane, any component can be nested within another. 48 Swing Features Contd Virtual Desktops and Compound Borders JDesktopPane and JInternalFrame classes can be used to create a virtual desktop. Compound borders are created by combining various border styles. Drag and Drop Facilitates transfer of data between Java applications as well as to native applications. Drag and drop support is available through the java.awt.dnd package. 49 Swing Features Contd Java 2D Using Java 2D API, one can: Create lines of any thickness Use different gradients and textures to fill shapes Move, rotate, scale and trim text and graphics Combine overlapping text and graphics Customized Dialogs and Standard Dialog classes Makes use of JOptionPane class Standard dialog classes available are: JFileChooser JColorChooser 50 Summary A Swing menu consists of a menubar, menuitems and menus. Trees are used to depict information in a hierarchical, vertical manner. In Swing, it is accomplished with the use of the JTree class. To display data in a tabular form we can make use of the JTable class in Swing. JProgressBar class is implemented to display graphical progress of a the task . Swing components work within the Model View Controller (MVC) model. 51 Summary Contd Characteristics common to all Swing components are content, visual appearance and behavior. The MVC model contains a set of classes and user interfaces for various platforms, which help in changing the “look and feel” of the component. KeyStroke handling, action objects, nested containers, virtual desktops, compound borders, drag and drop, Java 2D, customized dialogs, standard dialog classes and generic undo capabilities are some of the special features of Swing.
File đính kèm:
bai_giang_cong_nghe_java_chuong_11_basic_swing_component_tra.pdf