Bài giảng Phát triển phần mềm nguồn mở - Bài 4: Object Oriented Programming - Nguyễn Hữu Thể
Content
1. Class
2. Visibility
3. Properties & Methods
4. Getter & Setter
5. Create objects
6. Constructor
7. Destructor
8. Inheritance
9. Abstract class
10. Interfaces
11. Autoloading classes
12. Anonymous functions
13. Closures
14. Namespace
Bạn đang xem 20 trang mẫu của tài liệu "Bài giảng Phát triển phần mềm nguồn mở - Bài 4: Object Oriented Programming - Nguyễn Hữu Thể", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Phát triển phần mềm nguồn mở - Bài 4: Object Oriented Programming - Nguyễn Hữu Thể
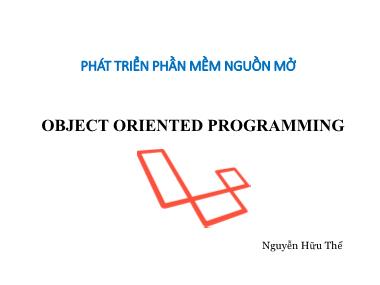
OBJECT ORIENTED PROGRAMMING Nguyễn Hữu Thể PHÁT TRIỂN PHẦN MỀM NGUỒN MỞ Content 1. Class 2. Visibility 3. Properties & Methods 4. Getter & Setter 5. Create objects 6. Constructor 7. Destructor 8. Inheritance 9. Abstract class 10. Interfaces 11. Autoloading classes 12. Anonymous functions 13. Closures 14. Namespace 2 Class 3 class ClassName { // Properties // Methods } Visibility (public, private, protected) Three levels: • public • private • protected By default, all class members are public. 4 Properties & Methods 5 class Person { private $name; //public, protected private $age; public function show(){ echo $this->name . " is " . $this->age . " years old!"; } } Create objects (Create a new instance of the class) Using the new keyword: new ClassName() For example: $person = new PerSon(); $person2 = new PerSon(); 6 Getter & Setter 7 class Person { private $name; private $age; public function getName(){ return $this->name; } public function setName($name){ $this->name = $name; } public function getAge(){ return $this->age; } public function setAge($age){ $this->age = $age; } } class Person { private String name; private int age; public String getName(){ return name; } public void setName(String name){ this.name = name; } public int getAge(){ return age; } public void setAge(int age){ this.age = age; } } PHP JAVA 8class Person{ private $name; private $age; public function getName(){ return $this->name; } public function setName($name){ $this->name = $name; } public function getAge(){ return $this->age; } public function setAge($age){ $this->age = $age; } public function show(){ echo $this->name . " is " . $this->age . " years old!"; } } $p = new Person(); $p->setName("Nguyễn Văn A"); $p->setAge(18); echo $p->getName() . " is " . $p->getAge() . " years old."; //echo "{$p->getName()} is {$p->getAge()} years old."; $p->show(); Ex: Person.php __set() method 9 class SetName { public function __set($variable, $value) { // echo $variable; echo "My " . $variable . " is " . $value . "\n"; } } $obj = new SetName (); $obj->Name = ‘Tom'; $obj->Name = ‘Jerry'; My Name is Tom My Name is Jerry __get() method 10 class GetName { public $type = 'chocolate'; public $choctype = array ( 'milk' => 0, 'dark' => 1, 'plain' => 2 ); public function wrap() { echo 'Its a wrap'; } public function __get($index) { echo '$choctype property with index of: ' . $index . ''; return $this->choctype [$index]; } } $candy = new GetName (); // set a non existant property echo 'Value of property is: ' . $candy->milk; $choctype property with index of: milk Value of property is: 0 Constructor 11 public function __construct($name, $age) { //... } public function __construct() { //... } Constructor 12 class Person{ private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function show(){ echo $this->name . " is " . $this->age . " years old!"; } } $p = new Person("Nguyễn Trần Lê", 18); $p->show(); Destructor Example: Using: 13 public function __destruct() { //... } public function __destruct() { echo "Bye bye!"; //... } $p = new Person("Nguyễn Trần Lê", 18); unset( $p ); // or exit: call __destruct() object $p->show(); // Object not found Inheritance 14 class ParentClass { public function myMethod() { // Method code here } } class ChildClass extends ParentClass { public function myMethod() { // For ChildClass objects, this method is called // instead of the parent class's MyMethod() } } Calling a parent method from a child method 15 parent::myMethod(); parent::show(); parent::__construct(); Example: Inheritance 16 require_once 'Person.php'; class Employee extends Person{ private $salary; public function __construct($name, $age, $salary){ parent::__construct($name, $age); // Call __construct() parent $this->salary = $salary; } public function getSalary(){ return $salary; } // Override public function show(){ parent::show(); echo " Salary: " . $this->salary; } public function display(){ echo " Name: " . $this->getName() . ""; echo " Age: " . $this->getAge() . ""; echo "Salary: " . $this->salary; } } $e = new Employee("Nguyễn Lê", 20, 200); $e->show(); Nguyễn Lê is 20 years old! Salary: 200 Visibility (Example 1) Property Visibility 17 class MyClass { public $public = 'Public'; protected $protected = 'Protected'; private $private = 'Private'; function printHello() { echo $this->public; echo $this->protected; echo $this->private; } } $obj = new MyClass(); echo $obj->public; // Works echo $obj->protected; // Fatal Error echo $obj->private; // Fatal Error $obj->printHello(); // Shows Public, Protected and Private Visibility (Example 2) Property Visibility 18 class MyClass2 extends MyClass { // We can redeclare the public and protected method, but not private public $public = 'Public2'; protected $protected = 'Protected2'; function printHello() { echo $this->public; echo $this->protected; echo $this->private; } } $obj2 = new MyClass2(); echo $obj2->public; // Works echo $obj2->protected; // Fatal Error echo $obj2->private; // Undefined $obj2->printHello(); // Shows Public2, Protected2, Undefined Visibility (Example 3) Method Visibility 19 class MyClass { // Declare a public constructor public function __construct() { } // Declare a public method public function MyPublic() { } // Declare a protected method protected function MyProtected() { } // Declare a private method private function MyPrivate() { } // This is public function Foo() { $this->MyPublic(); $this->MyProtected(); $this->MyPrivate(); } } $myclass = new MyClass; $myclass->MyPublic(); // Works $myclass->MyProtected(); // Fatal Error $myclass->MyPrivate(); // Fatal Error $myclass->Foo(); // Public, Protected and Private work Visibility (Example 4) Method Visibility 20 class MyClass2 extends MyClass { // This is public function Foo2() { $this->MyPublic(); $this->MyProtected(); $this->MyPrivate(); // Fatal Error } } $myclass2 = new MyClass2; $myclass2->MyPublic(); // Works $myclass2->Foo2(); // Public and Protected work, not Private Visibility (Example 5) Method Visibility 21 class Bar { public function test() { $this->testPrivate(); $this->testPublic(); } public function testPublic() { echo "Bar::testPublic\n"; } private function testPrivate() { echo "Bar::testPrivate\n"; } } class Foo extends Bar { public function testPublic() { echo "Foo::testPublic\n"; } private function testPrivate() { echo "Foo::testPrivate\n"; } } $myFoo = new Foo(); $myFoo->test(); // Bar::testPrivate // Foo::testPublic Abstract class An abstract class is a class that cannot be instantiated on its own. 22 abstract class Mathematics{ /*** child class must define these methods ***/ abstract protected function getMessage(); abstract protected function add($num); /** * method common to both classes **/ public function showMessage() { echo $this->getMessage(); } } Abstract class 23 require_once 'Mathematics.php'; class myMath extends Mathematics { protected function getMessage() { return "The anwser is: "; } public function add($num) { return $num + 2; } } // A new instance of myMath $myMath = new MyMath (); $myMath->showMessage (); echo $myMath->add (4); The anwser is: 6 Interfaces An interface declares one or more methods. Must be implemented by any class that implements the interface. 24 interface MyInterface { public function aMethod(); public function anotherMethod(); } class MyClass implements MyInterface { public function aMethod() { // (code to implement the method) } public function anotherMethod() { // (code to implement the method) } } interface Persistable { public function save(); public function load(); public function delete(); } require_once 'Persistable.php'; class Member implements Persistable { private $username; private $location; private $homepage; public function __construct( $username, $location, $homepage ) { $this->username = $username; $this->location = $location; $this->homepage = $homepage; } public function getUsername() { return $this->username; } public function getLocation() { return $this->location; } public function getHomepage() { return $this->homepage; } public function save() { echo "Saving member to database"; } public function load() { echo "Loading member from database"; } public function delete () { echo "Deleting member from database"; } } $m = new Member("Aha", "VN", "No page"); echo $m->getUsername() . ""; $m->load(); //... Aha Loading member from database class Topic implements Persistable { private $subject; private $author; private $createdTime; public function __construct( $subject, $author ) { $this->subject = $subject; $this->author = $author; $this->createdTime = time(); } public function showHeader() { $createdTimeString = date( 'l jS M Y h:i:s A', $this->createdTime ); $authorName = $this->author->getUsername(); echo "$this->subject (created on $createdTimeString by $authorName)"; } public function save() { echo "Saving topic to database"; } public function load() { echo "Loading topic from database"; } public function delete () { echo "Deleting topic from database"; } } require_once 'Member.php'; require_once 'Topic.php'; $aMember = new Member ( "TUI", "Viet Nam", "" ); echo $aMember->getUsername () . " lives in " . $aMember->getLocation () . ""; $aMember->save (); $aTopic = new Topic ( "PHP is Great", $aMember ); $aTopic->showHeader (); $aTopic->save (); TUI lives in Viet Nam Saving member to database PHP is Great (created on Saturday 17th Dec 2016 03:00:07 AM by TUI) Saving topic to database Test.php Autoloading classes Create a function called __autoload() near the start of your PHP application. PHP automatically calls your __autoload() function. 28 function __autoload($className) { require_once ("$className.php"); echo "Loaded $className.php"; } $member = new Member("TUI", "Viet Nam", "No page"); echo "Created object: "; print_r ( $member ); Loaded Member.php Created object: Member Object ( [username:Member:private] => TUI [location:Member:private] => Viet Nam [homepage:Member:private] => No page ) Anonymous functions Anonymous functions have no name. 29 // Assign an anonymous function to a variable $makeGreeting = function ($name, $timeOfDay) { return ('Good ' . $timeOfDay . ', ' . $name); }; // Call the anonymous function echo $makeGreeting ( 'Tom', 'morning' ) . ''; echo $makeGreeting ( 'Jerry', 'afternoon' ) . ''; Good morning, Tom Good afternoon, Jerry Closures A closure is an anonymous function that can access variables imported from the outside scope without using any global variables. 30 function myClosure($num) { return function ($x) use ($num) { return $num * $x; }; } $closure = myClosure ( 10 ); echo $closure ( 2 ); echo $closure ( 3 ); 20 30 Namespaces Namespaced code is defined using a singlenamespace keyword at the top of your PHP file. It must be the first command (with the exception ofdeclare) and no non-PHP code, HTML, or white-space 31 // define this code in the 'MyProject' namespace namespace MyProject; // ... code ... namespace foo; class Cat { static function says() { echo 'Meoow'; } } namespace bar; class Dog { static function says() { echo 'Ruff'; } } namespace other\animate; class Animal { static function breathes() { echo 'Air'; } } namespace test; include 'Cat.php'; include 'Dog.php'; include 'Animal.php'; use foo as cat; use bar as dog; //use other\animate; use other\animate as animal; echo cat\Cat::says (), ""; echo dog\Dog::says (), ""; echo animal\Animal::breathes (); //echo \other\animate\Animal::breathes (); Cat.php Dog.php Animal.php Test.php Result: Meoow Ruff Air
File đính kèm:
bai_giang_phat_trien_phan_mem_nguon_mo_chuong_4_object_orien.pdf